how to move from combine to async await
Generated on 8/6/2024
1 search
To move from Combine to async/await in Swift, you can follow these general steps:
-
Identify Combine Publishers: Locate the parts of your code where Combine publishers are used.
-
Replace Publishers with Async Functions: Convert these publishers into async functions. An async function in Swift is marked with the
async
keyword, and you use theawait
keyword to call these functions. -
Handle Asynchronous Operations: For operations like reading from disk or messaging another service, you can use the
await
keyword to wait for these operations to complete. This allows the CPU to handle other tasks while waiting. -
Use Continuations for Callbacks: If you have completion handlers, you can use
withCheckedContinuation
orwithCheckedThrowingContinuation
to convert them into async/await.
Here are some relevant segments from WWDC sessions that discuss async/await and concurrency in Swift:
-
A Swift Tour: Explore Swift’s features and design: This session covers the basics of writing concurrent code in Swift, including tasks, async/await, and actors. You can start learning about concurrency in Swift from the Concurrency chapter.
-
Explore Swift performance: This session explains how async functions are implemented and how they manage memory. You can learn about async functions starting from the Async functions chapter.
-
Migrate your app to Swift 6: This session provides practical examples of adopting Swift's concurrency features and handling shared mutable state. You can start from the Adopting concurrency features chapter.
-
Go further with Swift Testing: This session shows techniques for waiting on asynchronous conditions with Swift testing, which can be useful when converting tests from Combine to async/await. You can start from the Asynchronous conditions chapter.
By following these steps and referring to the mentioned sessions, you can effectively transition your code from Combine to async/await in Swift.
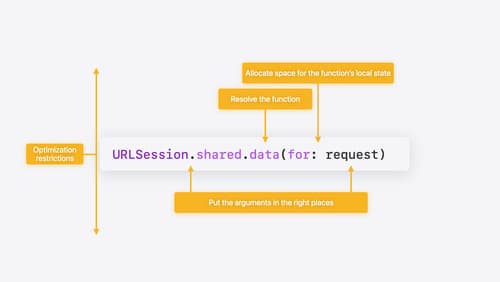
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
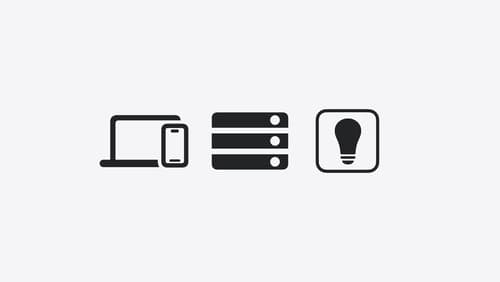
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
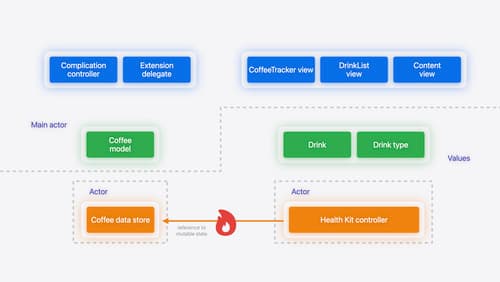
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
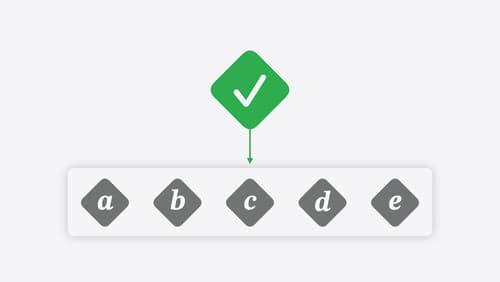
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.