How do I connect @Published in model to @Binding in a View?
Generated on 8/6/2024
1 search
To connect @Published
in a model to @Binding
in a SwiftUI view, you can follow these steps:
-
Define the Model with
@Published
Property: Create a model class that conforms toObservableObject
and use the@Published
property wrapper for the property you want to observe.class Pet: ObservableObject { @Published var rating: Int = 0 }
-
Create a View with
@Binding
: Define a SwiftUI view that takes a@Binding
to the property you want to bind.struct RatingView: View { @Binding var rating: Int var body: some View { VStack { Text("Rating: \(rating)") Button("Increase Rating") { rating += 1 } } } }
-
Bind the Model Property to the View: In your main view, create an instance of the model and pass a binding to the view.
struct ContentView: View { @StateObject private var pet = Pet() var body: some View { RatingView(rating: $pet.rating) } }
This setup ensures that changes in the model's @Published
property are reflected in the view, and vice versa.
For more detailed information, you can refer to the SwiftUI essentials session from WWDC 2024, which discusses how to use @Binding
to create a two-way reference to the state of another view.
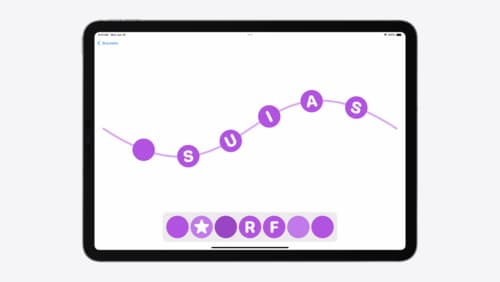
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
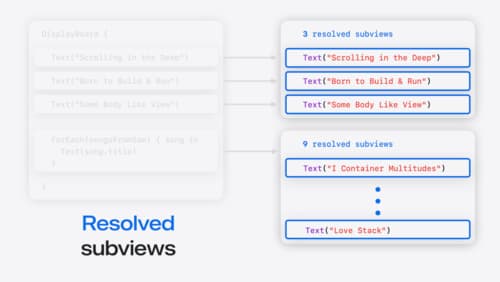
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
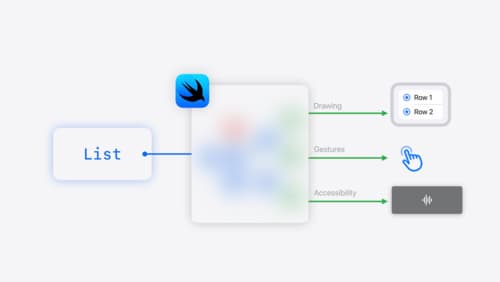
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.