How can I use TextKit 2 to draw custom background colors in specific parts of an attributed string?
Generated on 8/6/2024
1 search
To draw custom background colors in specific parts of an attributed string using TextKit 2, you can leverage the new text attribute protocol introduced alongside the text renderer in iOS 18 and aligned releases. Here’s a step-by-step guide on how to achieve this:
-
Implement the Text Attribute Protocol: By implementing this protocol, you can pass data from your text to your text renderer. This allows you to define custom attributes for specific parts of your text.
-
Apply the Custom Attribute: Use the custom attribute text modifier to mark the specific parts of your text that you want to customize. For example, you can create a custom emphasis attribute to highlight certain words.
-
Check for the Attribute in the Draw Method: In your draw method, iterate over the flattened runs of your layout and check for the presence of your custom attribute. If the attribute is present, you can then apply your custom background color.
Here’s a brief example based on the context provided:
struct CustomEmphasisAttribute: TextAttribute {
// No need for member variables if it's just a marker
}
// Applying the custom attribute
let attributedString = NSMutableAttributedString(string: "This is a sample text with custom background.")
attributedString.addAttribute(CustomEmphasisAttribute.self, value: CustomEmphasisAttribute(), range: NSRange(location: 10, length: 6))
// In the draw method
for run in layoutManager.textStorage?.runs ?? [] {
if let _ = run[CustomEmphasisAttribute.self] {
// Apply custom background color
context.setFillColor(UIColor.yellow.cgColor)
context.fill(run.boundingRect)
}
}
For more detailed information, you can refer to the session Create custom visual effects with SwiftUI (18:31).
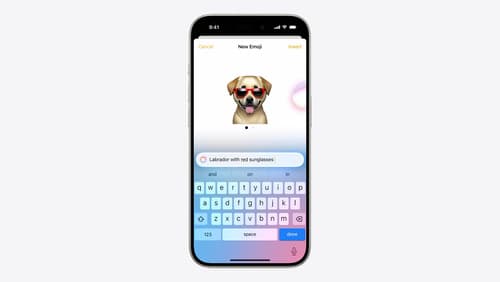
Bring expression to your app with Genmoji
Discover how to bring Genmoji to life in your app. We’ll go over how to render, store, and communicate text that includes Genmoji. If your app features a custom text engine, we’ll also cover techniques for adding support for Genmoji.
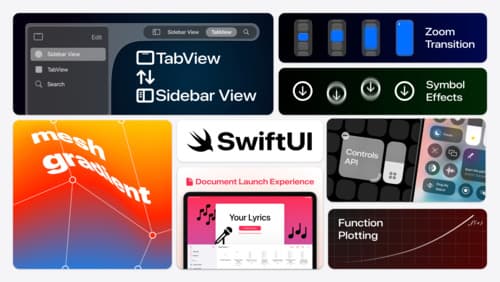
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
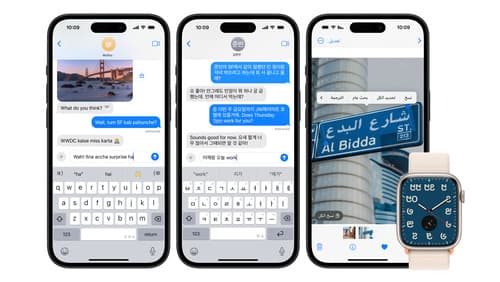
Build multilingual-ready apps
Ensure your app works properly and effectively for multilingual users. Learn best practices for text input, display, search, and formatting. Get details on typing in multiple languages without switching between keyboards. And find out how the latest advances in the String Catalog can make localization even easier.
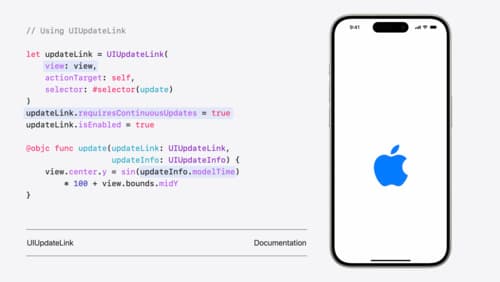
What’s new in UIKit
Explore everything new in UIKit, including tab and document launch experiences, transitions, and text and input changes. We’ll also discuss better-than-ever interoperability between UIKit and SwiftUI animations and gestures, as well as general improvements throughout UIKit.
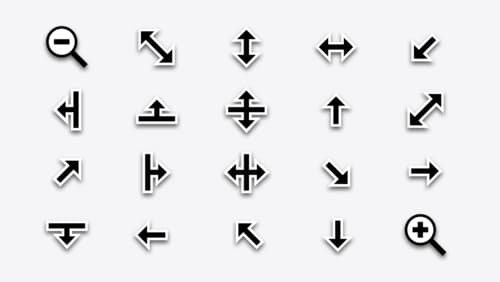
What’s new in AppKit
Discover the latest advances in Mac app development. Get an overview of the new features in macOS Sequoia, and how to adopt them in your app. Explore new ways to integrate your existing code with SwiftUI. Learn about the improvements made to numerous AppKit controls, like toolbars, menus, text input, and more.
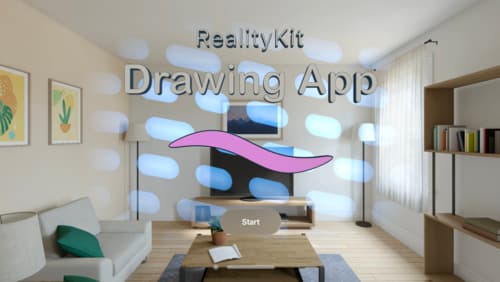
Build a spatial drawing app with RealityKit
Harness the power of RealityKit through the process of building a spatial drawing app. As you create an eye-catching spatial experience that integrates RealityKit with ARKit and SwiftUI, you’ll explore how resources work in RealityKit and how to use features like low-level mesh and texture APIs to achieve fast updates of the users’ brush strokes.
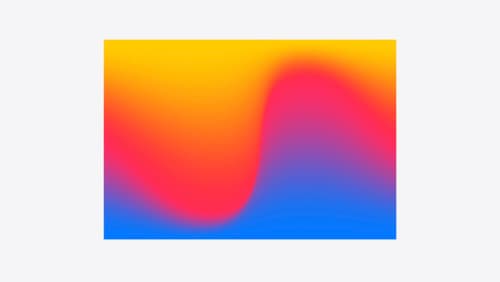
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
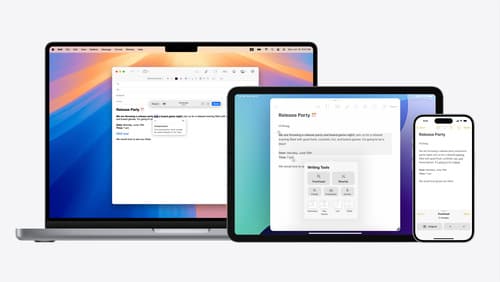
Get started with Writing Tools
Learn how Writing Tools help users proofread, rewrite, and transform text in your app. Get the details on how Writing Tools interact with your app so users can refine what they have written in any text view. Understand how text is retrieved and processed, and how to support Writing Tools in custom text views.