When using Swift concurrency, how can I call async code from a synchronous context and wait for the result?
Asked on 08/06/2024
1 search
To call async code from a synchronous context and wait for the result in Swift, you can use the withCheckedContinuation
or withCheckedThrowingContinuation
functions. These functions allow you to bridge between synchronous and asynchronous code by creating a continuation that you can resume once the asynchronous operation completes.
Here's a brief example of how you might use withCheckedContinuation
:
func performAsyncOperation() async -> String {
// Simulate an async operation
return "Async result"
}
func callAsyncFromSync() -> String {
var result: String = ""
let semaphore = DispatchSemaphore(value: 0)
Task {
result = await performAsyncOperation()
semaphore.signal()
}
semaphore.wait()
return result
}
In this example, performAsyncOperation
is an asynchronous function. The callAsyncFromSync
function creates a DispatchSemaphore
to wait for the asynchronous operation to complete. Inside a Task
, it calls the async function and signals the semaphore once the result is obtained. The synchronous function then waits for the semaphore to be signaled before returning the result.
For more detailed information on using continuations in Swift, you can refer to the session Go further with Swift Testing (24:51), which discusses techniques for waiting on asynchronous conditions with Swift testing, including the use of withCheckedContinuation
and withCheckedThrowingContinuation
.
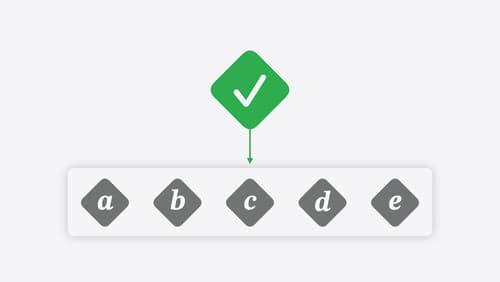
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
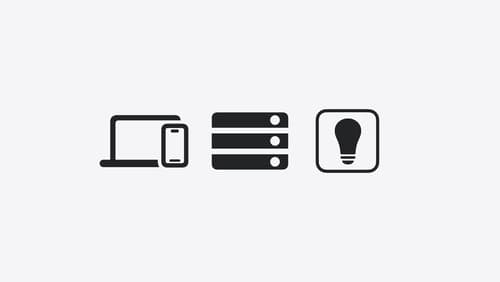
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
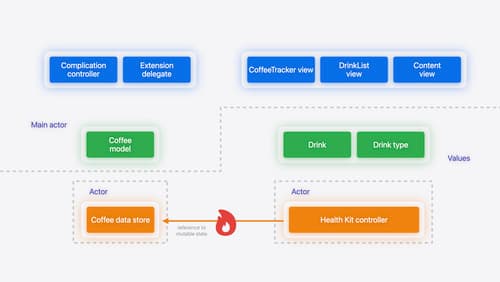
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.