how to create anotation like @MainActor
Generated on 8/7/2024
1 search
To create an annotation like @MainActor
in Swift, you can use the @MainActor
attribute to indicate that a particular piece of code should be executed on the main actor. This is particularly useful for UI-related code that must run on the main thread to avoid concurrency issues.
Here are some key points from the WWDC session "Migrate your app to Swift 6" that explain how to use @MainActor
:
-
Annotating Methods and Properties: You can annotate methods or properties to indicate that they should be executed on the main actor. For example:
@MainActor func updateUI() { // UI update code }
-
Assume Isolated: If you know that a method will always be called on the main actor, you can use
MainActor.assumeIsolated
to inform the compiler:MainActor.assumeIsolated { // Code that assumes it's running on the main actor }
This does not start a new task but tells Swift that the code is already running on the main actor. This can help avoid unnecessary context switching.
-
Protocol Conformance: You can also annotate entire protocols to indicate that all conforming types should be isolated to the main actor:
@MainActor protocol MyMainActorProtocol { func performTask() }
-
Implicit Isolation: In Swift 6, many delegates and protocols, such as
SwiftUI.View
, are implicitly isolated to the main actor. This means you might not need to add@MainActor
annotations explicitly if the protocol or delegate already guarantees main actor isolation.
For more detailed information, you can refer to the session Migrate your app to Swift 6 (19:29).
Relevant Sessions
These sessions provide comprehensive guidance on using @MainActor
and other concurrency features in Swift 6.
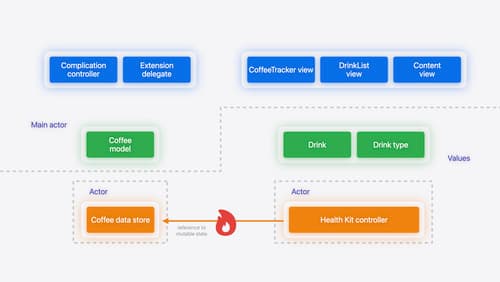
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
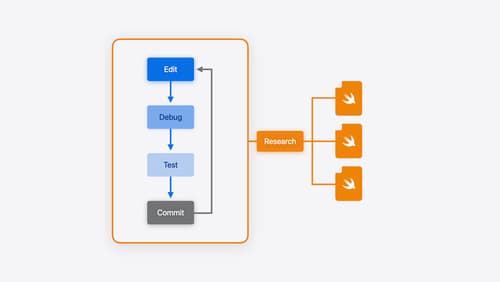
Xcode essentials
Edit, debug, commit, repeat. Explore the suite of tools in Xcode that help you iterate quickly when developing apps. Discover tips and tricks to help optimize and boost your development workflow.
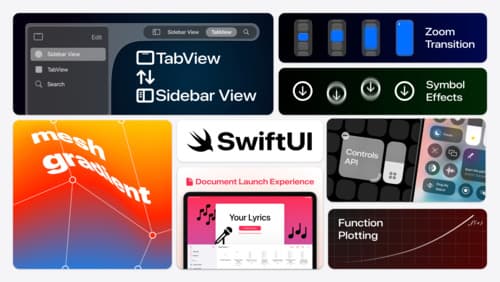
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.