How can I use a CollectionView in Swiftui
Generated on 8/7/2024
1 search
To use a CollectionView
in SwiftUI, you can leverage the List
and ForEach
views, which are powerful tools for displaying collections of data. Here are some key points and examples from the WWDC sessions:
-
Using
List
with a Collection Parameter:- You can initialize a
List
with a collection parameter, which is a convenience for creating aForEach
view. TheForEach
view generates views for each element in a collection and provides those to its container. - Example from the session SwiftUI essentials:
List(pets) { pet in PetView(pet: pet) }
- You can initialize a
-
Using
ForEach
for More Flexibility:- The
ForEach
view can be used to iterate over a collection and generate views for each item. This can be nested within other views for more complex layouts. - Example from the session Demystify SwiftUI containers:
ForEach(songIdeas) { song in Text(song.title) }
- The
-
Creating Sections in a List:
- You can use the
Section
view to organize data into sections within aList
. This is useful for creating more structured and organized layouts. - Example from the session Demystify SwiftUI containers:
List { Section(header: Text("My Songs")) { ForEach(mySongs) { song in Text(song.title) } } Section(header: Text("Recommended Songs")) { ForEach(recommendedSongs) { song in Text(song.title) } } }
- You can use the
-
Using
LazyVGrid
for Grid Layouts:- For grid layouts, you can use
LazyVGrid
to create a flexible grid structure. - Example from the session Migrate your TVML app to SwiftUI:
LazyVGrid(columns: [GridItem(.flexible()), GridItem(.flexible()), GridItem(.flexible()), GridItem(.flexible())], spacing: 40) { ForEach(items) { item in ItemView(item: item) } }
- For grid layouts, you can use
These examples illustrate how you can use List
, ForEach
, Section
, and LazyVGrid
to create and manage collection views in SwiftUI. For more detailed information, you can refer to the sessions mentioned above.
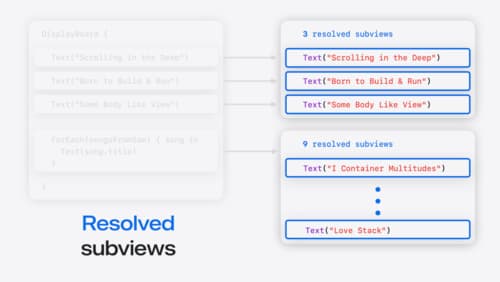
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
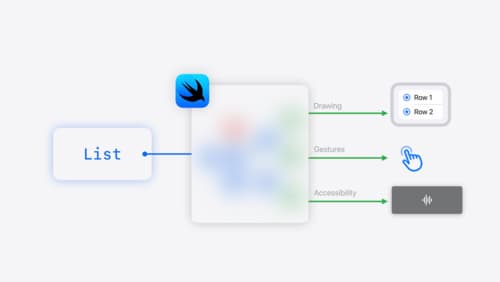
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
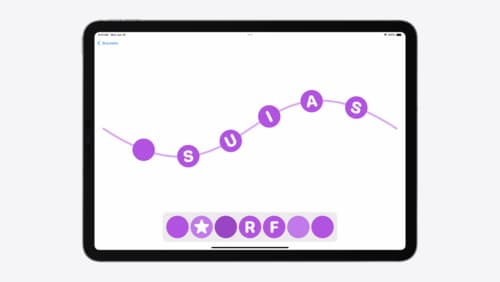
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
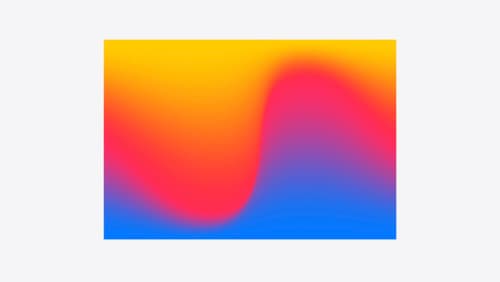
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
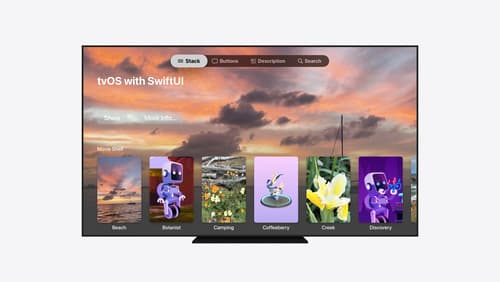
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.