whats generics
Generated on 8/7/2024
1 search
Generics in Swift allow you to write flexible and reusable code by enabling you to define functions, types, and protocols that can work with any type. Here are some key points about generics from the WWDC sessions:
-
Basic Concept:
- Generics enable you to write functions and types that can operate on any type, specified as a placeholder. For example, a generic function might look like this:
Here,func execute<T>(item: T) { // Function body }
T
is a placeholder for any type.
- Generics enable you to write functions and types that can operate on any type, specified as a placeholder. For example, a generic function might look like this:
-
Constraints:
- You can add constraints to generics to specify that the placeholder type must conform to certain protocols. For example, you might require that a type conforms to the
Hashable
protocol:
This ensures that the function can only be called with types that conform tofunc uniqued<T: Hashable>(items: [T]) -> [T] { // Function body }
Hashable
.
- You can add constraints to generics to specify that the placeholder type must conform to certain protocols. For example, you might require that a type conforms to the
-
Noncopyable Generics:
- Swift 6 introduces noncopyable generics, which allow you to define generics that do not require the types to be copyable. This is useful for types that manage resources and should not be copied.
- For example, you can define a generic function that works with noncopyable types by using the
~copyable
constraint:
This broadens the types permitted by the function to include noncopyable types.func execute<T: ~copyable>(item: T) { // Function body }
-
Embedded Swift:
- Generics are fully supported in Embedded Swift, allowing you to write efficient and reusable code even in constrained environments. The compiler can specialize generic functions to avoid runtime overhead.
For more detailed information, you can refer to the following sessions:
- Consume noncopyable types in Swift
- A Swift Tour: Explore Swift’s features and design
- Go small with Embedded Swift
These sessions provide a comprehensive overview of generics, their constraints, and their applications in different contexts.
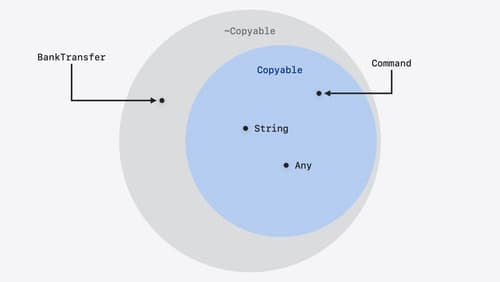
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.
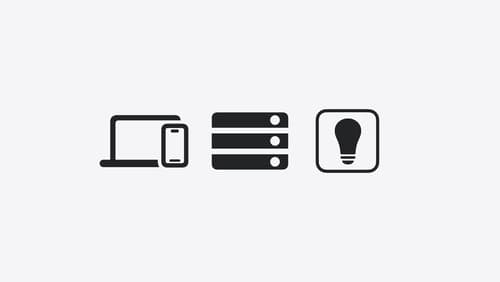
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
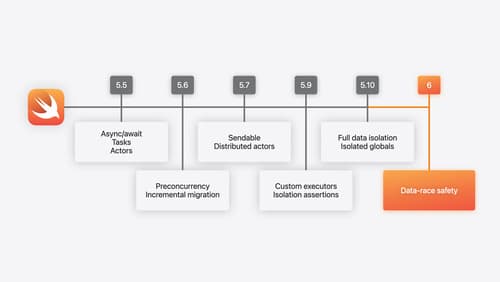
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
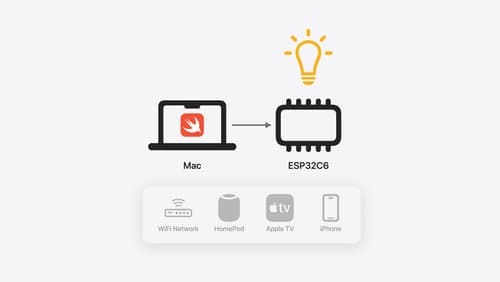
Go small with Embedded Swift
Embedded Swift brings the safety and expressivity of Swift to constrained environments. Explore how Embedded Swift runs on a variety of microcontrollers through a demonstration using an off-the-shelf Matter device. Learn how the Embedded Swift subset packs the benefits of Swift into a tiny footprint with no runtime, and discover plenty of resources to start your own Embedded Swift adventure.