How can I open my app when an indexed entity is tapped in Spotlight?
Generated on 8/7/2024
1 search
To open your app when an indexed entity is tapped in Spotlight, you need to follow these steps:
-
Conform to the
IndexedEntity
Protocol: First, ensure that your entity (e.g., a trail entity) conforms to the newIndexedEntity
protocol. This allows your entity to be indexed by Spotlight. -
Index Your Entities: In your app's initialization method, index all the entities using
CSSearchableIndex
. This can be done by callingindexAppEntities
onCSSearchableIndex
. -
Implement an Intent: Create an intent that can handle the entity as a parameter. For example, an
OpenTrailIntent
that accepts a trail entity. -
Donate the Entity to Spotlight: Once your entities are indexed, they will appear in Spotlight search results. When a user taps on a search result, the associated intent will be triggered, opening your app to the relevant content.
Here is a brief example of how this can be implemented:
// Conform to IndexedEntity
struct Trail: IndexedEntity {
var id: String
var name: String
// Other properties
// Implement the required methods
static var entityIdentifier: String {
return "com.example.trail"
}
var displayRepresentation: DisplayRepresentation {
return DisplayRepresentation(title: name)
}
var attributeSet: CSSearchableItemAttributeSet {
let attributes = CSSearchableItemAttributeSet(itemContentType: kUTTypeItem as String)
attributes.title = name
// Add other attributes
return attributes
}
}
// Index the entities
func indexTrails() {
let trails = dataManager.getAllTrails()
CSSearchableIndex.default().indexAppEntities(trails)
}
// Create an intent
class OpenTrailIntent: INIntent {
@NSManaged var trail: Trail?
}
// Donate the entity to Spotlight
func donateTrailToSpotlight(trail: Trail) {
let intent = OpenTrailIntent()
intent.trail = trail
let interaction = INInteraction(intent: intent, response: nil)
interaction.donate { error in
if let error = error {
print("Failed to donate interaction: \(error)")
}
}
}
For more detailed information, you can refer to the session What’s new in App Intents (02:35).
Relevant Sessions
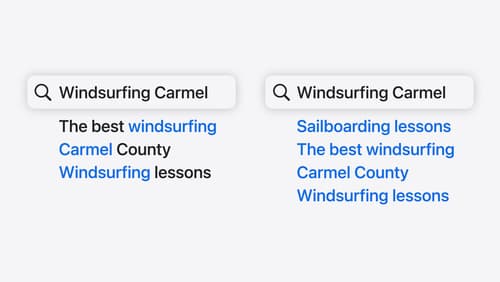
Support semantic search with Core Spotlight
Learn how to provide semantic search results in your app using Core Spotlight. Understand how to make your app’s content available in the user’s private, on-device index so people can search for items using natural language. We’ll also share how to optimize your app’s performance by scheduling indexing activities. To get the most out of this session, we recommend first checking out Core Spotlight documentation on the Apple Developer website.
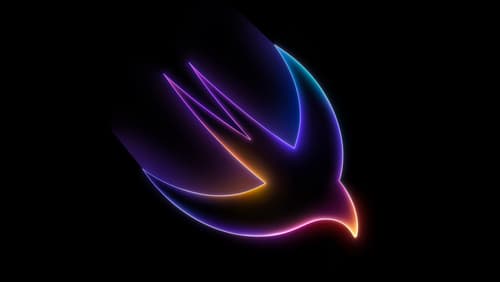
Platforms State of the Union
Discover the newest advancements on Apple platforms.
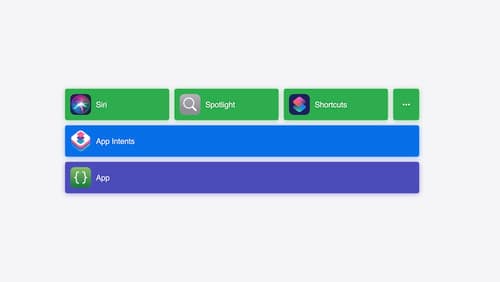
Bring your app’s core features to users with App Intents
Learn the principles of the App Intents framework, like intents, entities, and queries, and how you can harness them to expose your app’s most important functionality right where people need it most. Find out how to build deep integration between your app and the many system features built on top of App Intents, including Siri, controls and widgets, Apple Pencil, Shortcuts, the Action button, and more. Get tips on how to build your App Intents integrations efficiently to create the best experiences in every surface while still sharing code and core functionality.
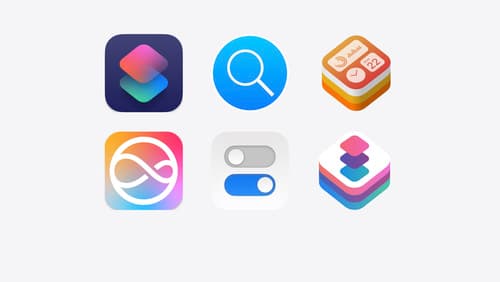
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.