How to do testing in SwiftUI?
Generated on 8/7/2024
1 search
To do testing in SwiftUI, you can leverage the new Swift Testing framework introduced by Apple. Here are some key points and steps to get you started:
-
Creating a Test:
- Start by creating a new file in your test folder.
- Import the testing framework and your app.
- Write a function for your test and add the
@Test
attribute to it. This marks the function as a test case.
import TestingFramework import MyApp @Test func testPlantingRoses() { let plant = Plant(type: .rose) XCTAssertEqual(plant.style, .grafting) }
-
Using Macros:
- Use the
expect
macro to verify results. This macro allows you to write simple or complex Swift expressions to check the expected outcomes.
@Test func testPlantingRoses() { let plant = Plant(type: .rose) expect(plant.style == .grafting) }
- Use the
-
Organizing Tests with Tags:
- Swift Testing allows you to use tags to organize and filter your tests. You can add tags to your test functions to group related tests together.
@Test(tags: ["planting"]) func testPlantingRoses() { let plant = Plant(type: .rose) expect(plant.style == .grafting) }
-
Parameterized Tests:
- You can create parameterized tests to run the same logic with different inputs. This helps avoid duplicating test code.
@Test func testPlantingStyles() { let styles: [PlantStyle] = [.grafting, .budding, .cutting] for style in styles { let plant = Plant(type: .rose, style: style) expect(plant.style == style) } }
-
Concurrency and Async/Await:
- Swift Testing integrates seamlessly with Swift concurrency, supporting
async/await
and actor isolation. This is useful for testing asynchronous code.
@Test func testAsyncPlanting() async { let plant = await Plant(type: .rose) expect(plant.style == .grafting) }
- Swift Testing integrates seamlessly with Swift concurrency, supporting
For more detailed information and examples, you can refer to the following sessions from WWDC 2024:
- Meet Swift Testing (09:47)
- What’s new in Xcode 16 (12:55)
- Platforms State of the Union (33:01)
These sessions cover the new Swift Testing framework, its features, and how to integrate it into your SwiftUI projects.
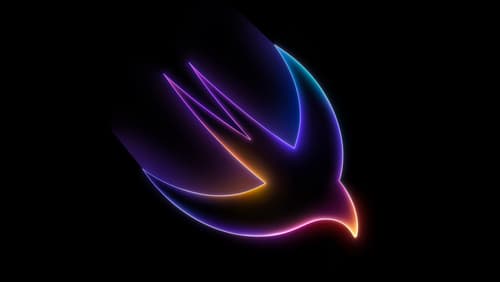
Platforms State of the Union
Discover the newest advancements on Apple platforms.
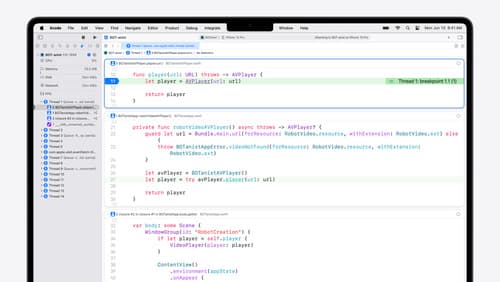
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.
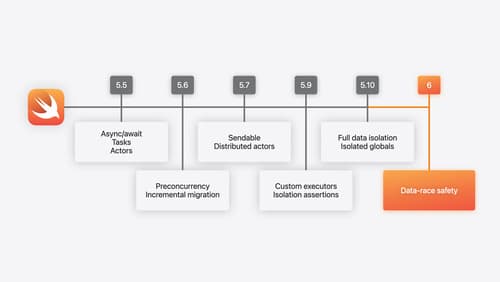
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
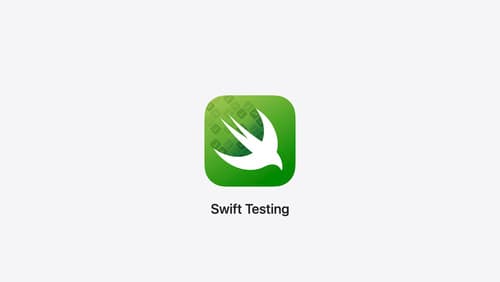
Meet Swift Testing
Introducing Swift Testing: a new package for testing your code using Swift. Explore the building blocks of its powerful new API, discover how it can be applied in common testing workflows, and learn how it relates to XCTest and open source Swift.