How to write an initializer?:
Generated on 8/7/2024
1 search
To write an initializer in Swift, you can follow these general steps:
-
Define the Initializer: Use the
init
keyword to define an initializer. You can create multiple initializers with different parameters. -
Set Initial Values: Inside the initializer, set the initial values for the properties of the class or struct.
-
Optional Initializers: You can create failable initializers that return
nil
if initialization fails.
Here are some examples from the WWDC sessions:
Deep Copy Initializer
In the session "Consume noncopyable types in Swift," an example of a deep copy initializer is provided. This initializer recreates an object and everything the object points to recursively, ensuring no shared references:
class Player {
var icon: Icon
init(icon: Icon) {
self.icon = icon
}
// Deep copy initializer
init(copying player: Player) {
self.icon = Icon(copying: player.icon)
}
}
Failable Initializer
In the session "What’s new in Swift," a failable initializer is discussed. This type of initializer returns an optional, which can be nil
if initialization fails:
struct File {
let name: String
init?(name: String) {
guard !name.isEmpty else {
return nil
}
self.name = name
}
}
Lazy Initialization
In the session "Migrate your app to Swift 6," lazy initialization of global variables is explained. This ensures that the variable is initialized only when it is first accessed:
class Logger {
static let shared = Logger()
private init() {
// Initialization code
}
}
Using ViewBuilder in SwiftUI
In the session "Demystify SwiftUI containers," an initializer using the @ViewBuilder
attribute is shown. This allows for the construction of views using a trailing closure:
struct ContentView: View {
var body: some View {
DisplayBoard {
Text("Hello, World!")
}
}
}
struct DisplayBoard<Content: View>: View {
let content: Content
init(@ViewBuilder content: () -> Content) {
self.content = content()
}
var body: some View {
VStack {
content
}
}
}
For more detailed information, you can refer to the following sessions:
- Consume noncopyable types in Swift
- What’s new in Swift
- Migrate your app to Swift 6
- Demystify SwiftUI containers
These examples should give you a good starting point for writing initializers in Swift.
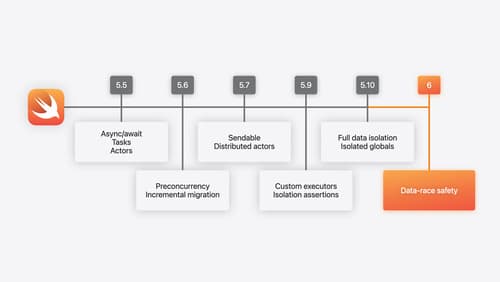
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
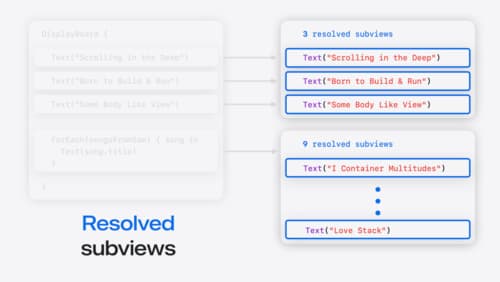
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
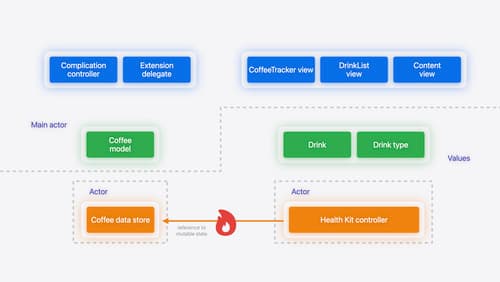
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
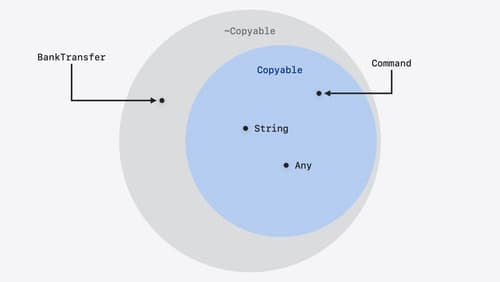
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.
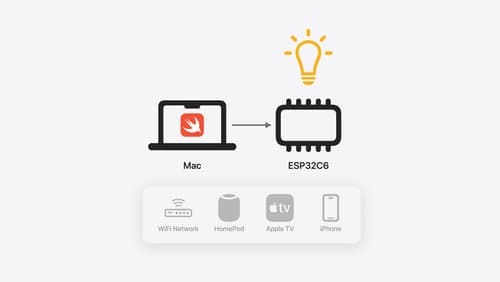
Go small with Embedded Swift
Embedded Swift brings the safety and expressivity of Swift to constrained environments. Explore how Embedded Swift runs on a variety of microcontrollers through a demonstration using an off-the-shelf Matter device. Learn how the Embedded Swift subset packs the benefits of Swift into a tiny footprint with no runtime, and discover plenty of resources to start your own Embedded Swift adventure.
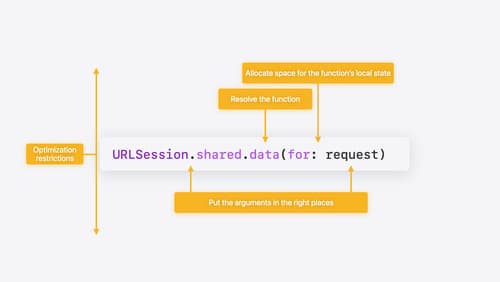
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.