how public properties within internal class or struct are accessible while the class or struct itself remains accessible only within the same module.
Generated on 8/7/2024
1 search
In Swift, you can have public properties within an internal class or struct, making the properties accessible from any module while the class or struct itself remains accessible only within the same module. This is achieved through Swift's access control levels.
Here's a brief overview of the relevant access control levels in Swift:
- public: Accessible from any module.
- internal: Accessible only within the same module.
- private: Accessible only within the same file.
When you declare a class or struct as internal
(which is the default if no access level is specified), it means that the class or struct can only be accessed within the same module. However, you can still declare its properties as public
, making those properties accessible from any module.
For example:
internal class MyClass {
public var myProperty: String
internal var anotherProperty: Int
init(myProperty: String, anotherProperty: Int) {
self.myProperty = myProperty
self.anotherProperty = anotherProperty
}
}
In this example, MyClass
is internal
, so it can only be accessed within the same module. However, myProperty
is public
, so it can be accessed from any module, while anotherProperty
remains internal
.
For more details on access control in Swift, you can refer to the session A Swift Tour: Explore Swift’s features and design from WWDC 2024.
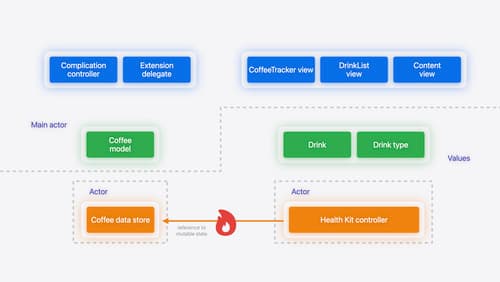
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
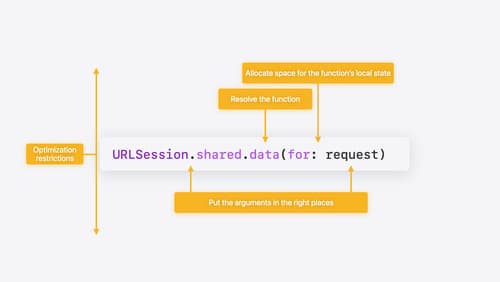
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
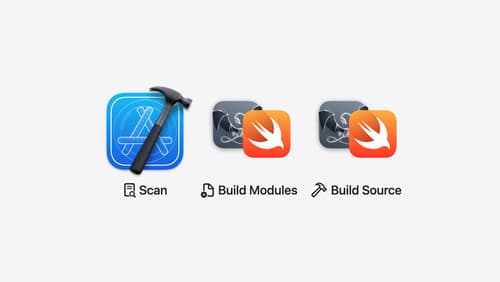
Demystify explicitly built modules
Explore how builds are changing in Xcode 16 with explicitly built modules. Discover how modules are used to build your code, how explicitly built modules improve transparency in compilation tasks, and how you can optimize your build by sharing modules across targets.
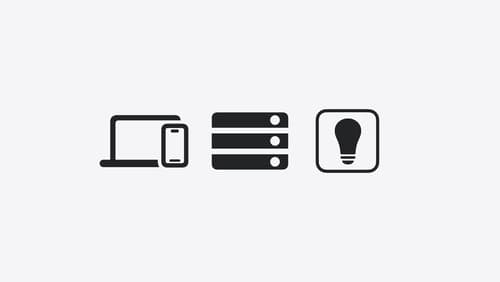
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.