How to properly run code on GCD?
Generated on 8/7/2024
1 search
To properly run code on Grand Central Dispatch (GCD), you need to understand the concurrency architecture and how to manage different queues effectively. Here are some key points from the WWDC session "Migrate your app to Swift 6" that can help:
-
Concurrency Architecture:
- The original app had multiple concurrent queues, including the main queue for UI and model work, a dispatch queue for background work, and arbitrary queues for certain callbacks (e.g., from HealthKit).
- By adopting Swift concurrency, the architecture was simplified. UI views and models were set to run on the main actor, while background operations were performed on dedicated actors. This setup ensures that the concurrency architecture is as clear and organized as the type architecture.
-
Main Actor and Background Actors:
- The main actor is used for UI-related tasks to ensure they run on the main thread.
- Background actors handle operations that do not need to run on the main thread, improving performance and avoiding UI blocking.
-
Thread Safety and Data Races:
- Swift concurrency helps in making guarantees explicit, reducing the risk of data races. For example, marking a delegate as
@MainActor
ensures that its methods and property accesses are made on the main thread. - Enabling strict concurrency checking in your target's build settings can help identify potential race conditions.
- Swift concurrency helps in making guarantees explicit, reducing the risk of data races. For example, marking a delegate as
-
Handling Callbacks:
- Always check the concurrency guarantees of callbacks. Some callbacks guarantee execution on the main thread, while others may run on arbitrary threads or queues.
- If a callback does not guarantee the thread it runs on, you may need to redispatch it to the appropriate queue or actor.
For more detailed information, you can refer to the session Migrate your app to Swift 6 (20:39).
Relevant Sessions
- Migrate your app to Swift 6
- What’s new in Xcode 16 - Thread performance checker
These sessions provide insights into managing concurrency and ensuring thread safety in your applications.
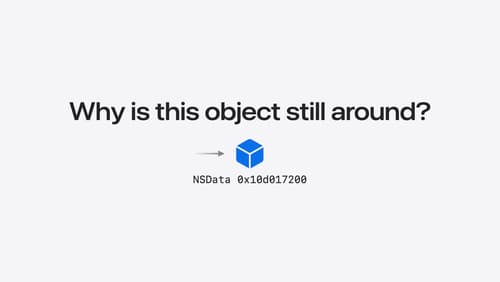
Analyze heap memory
Dive into the basis for your app’s dynamic memory: the heap! Explore how to use Instruments and Xcode to measure, analyze, and fix common heap issues. We’ll also cover some techniques and best practices for diagnosing transient growth, persistent growth, and leaks in your app.
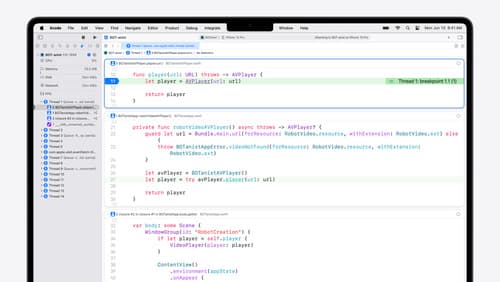
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.
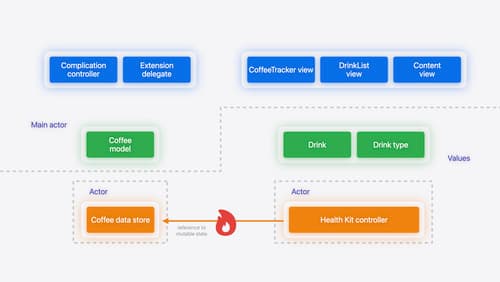
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.