tell me about the differences between ARC and ownership in Swift
Generated on 8/8/2024
1 search
Automatic Reference Counting (ARC) and ownership in Swift are both mechanisms for managing memory, but they operate at different levels and have distinct purposes.
Automatic Reference Counting (ARC)
ARC is a memory management feature in Swift that automatically keeps track of the number of references to each instance of a class. When the reference count of an object drops to zero, the object is deallocated. This process is predictable and helps in managing memory efficiently. However, one challenge with ARC is the potential for strong reference cycles, which can prevent objects from being deallocated. To break these cycles, Swift provides weak and unowned references:
- Weak References: These do not increase the reference count of the object they refer to and are always optional. They become
nil
when the object they reference is deallocated. This is useful for breaking reference cycles but comes with some overhead due to the need for additional storage to manage the weak reference. - Unowned References: These also do not increase the reference count but are non-optional. They assume the referenced object will always be in memory while the unowned reference exists. This is more efficient but can lead to crashes if the object is deallocated unexpectedly.
For more details, you can refer to the session A Swift Tour: Explore Swift’s features and design (13:24).
Ownership in Swift
Ownership in Swift is a broader concept that encompasses how values are managed and transferred within the language. It is a key part of Swift's memory safety model and involves three main kinds of ownership interactions:
- Consuming a Value: This means transferring ownership of a value from one place to another, such as when assigning a value to a variable.
- Mutating a Value: This involves changing the value while maintaining ownership.
- Borrowing a Value: This means temporarily using a value without taking ownership, ensuring that the value is not mutated or consumed by others during the borrow.
Ownership is crucial for managing both value types and reference types in Swift. For example, when a container owns an array, it is responsible for managing the array's buffer, including retaining and releasing it as needed.
For more details, you can refer to the session Explore Swift performance (13:46).
Summary
- ARC: Manages memory for reference types by keeping track of reference counts and deallocating objects when their count drops to zero. It uses weak and unowned references to handle reference cycles.
- Ownership: A broader concept that includes consuming, mutating, and borrowing values, ensuring memory safety and efficient management of both value and reference types.
These mechanisms work together to provide a robust and efficient memory management system in Swift.
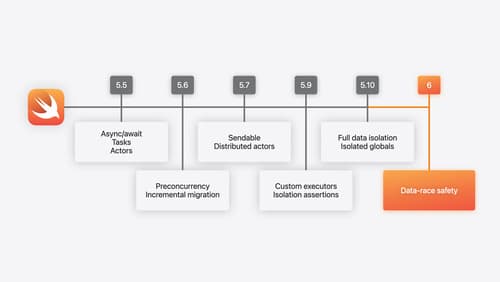
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
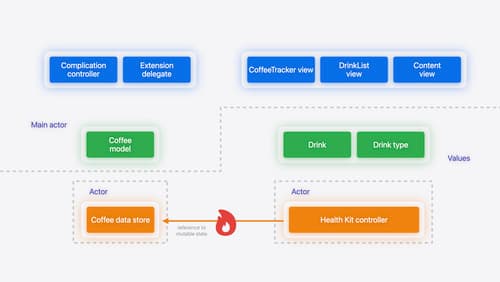
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
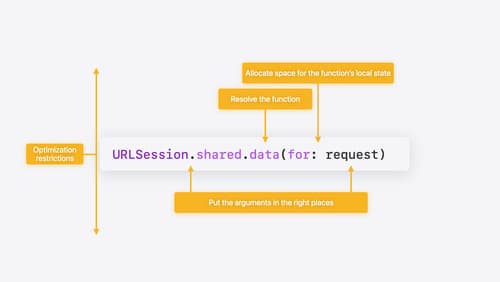
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
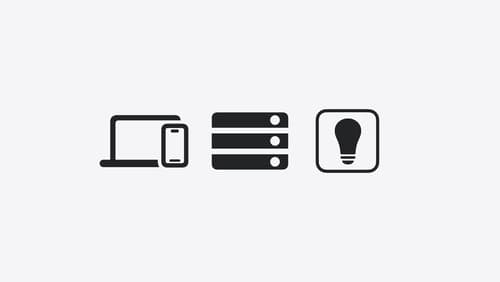
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
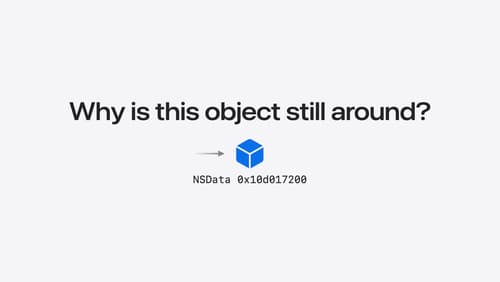
Analyze heap memory
Dive into the basis for your app’s dynamic memory: the heap! Explore how to use Instruments and Xcode to measure, analyze, and fix common heap issues. We’ll also cover some techniques and best practices for diagnosing transient growth, persistent growth, and leaks in your app.