How to use @Binding
Generated on 8/8/2024
2 searches
To use @Binding
in SwiftUI, you can follow these steps:
-
Define a State Property: In the parent view, define a state property that will hold the value you want to bind.
-
Pass the Binding: Pass a binding of this state property to the child view.
-
Use the Binding: In the child view, use the
@Binding
property wrapper to create a two-way reference to the state property in the parent view.
Here's a simple example to illustrate:
Parent View
import SwiftUI
struct ParentView: View {
@State private var rating: Int = 0
var body: some View {
VStack {
Text("Rating: \(rating)")
RatingView(rating: $rating)
}
}
}
Child View
import SwiftUI
struct RatingView: View {
@Binding var rating: Int
var body: some View {
HStack {
Button(action: {
rating -= 1
}) {
Text("-")
}
Text("\(rating)")
Button(action: {
rating += 1
}) {
Text("+")
}
}
}
}
In this example:
- The
ParentView
has a state propertyrating
. - The
RatingView
receives a binding to this state property via$rating
. - The
RatingView
can then read and modify therating
value, and these changes will be reflected in theParentView
.
For more detailed information, you can refer to the SwiftUI essentials session from WWDC 2024.
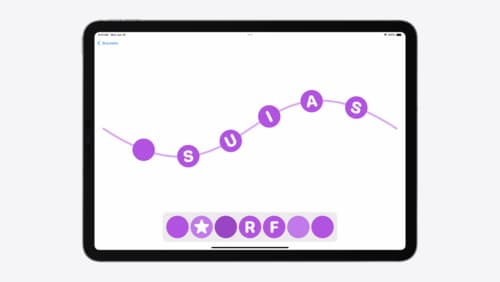
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
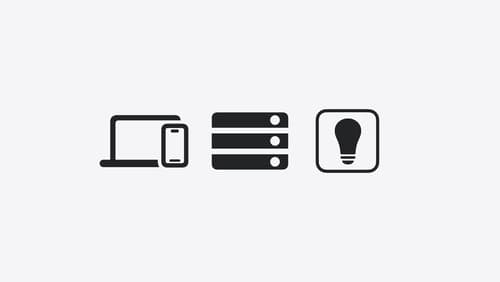
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
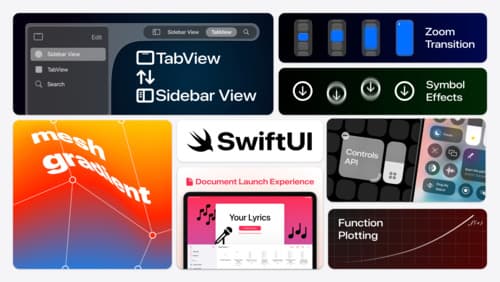
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
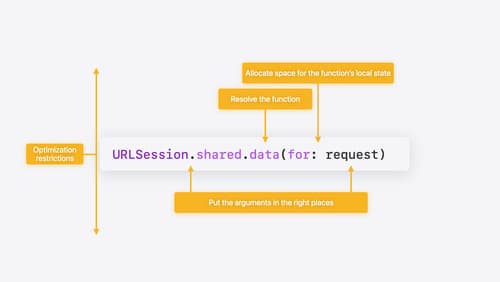
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
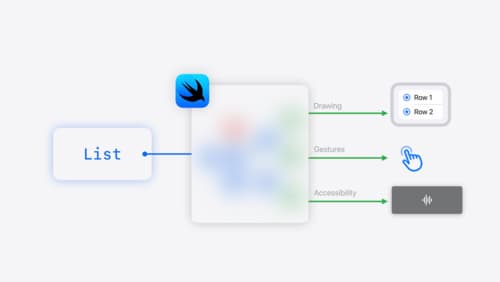
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
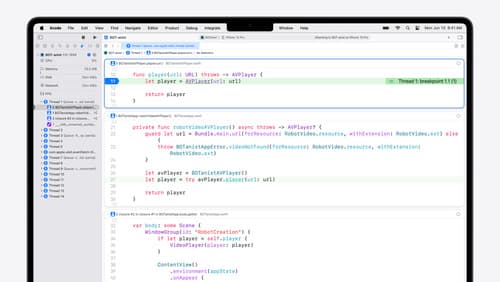
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.