How does cooperative thread pool work?
Generated on 8/8/2024
1 search
The cooperative thread pool in Swift is designed to manage concurrency efficiently by allowing tasks to yield the CPU when they are waiting for asynchronous operations to complete. This is achieved through the use of async functions and tasks, which are the fundamental units of concurrency in Swift.
Here are some key points about how cooperative thread pools work in Swift:
-
Tasks and Async Functions: Tasks represent independent concurrent execution contexts and are lightweight, allowing for the creation of many tasks. Async functions are split into multiple partial functions at runtime, which handle different parts of the function around suspension points (e.g.,
await
). -
Memory Management: Async functions allocate memory on an async stack, which is different from the C stack. This stack is managed in slabs, and memory is allocated from these slabs as needed. If a slab is full, a new one is allocated.
-
Suspension and Resumption: When a task performs an asynchronous operation, it suspends and yields the CPU to other tasks. This allows other tasks to run while the original task is waiting for the async operation to complete. Once the async operation completes, the task resumes execution.
-
Actors: Actors in Swift help manage shared mutable state by serializing access to their state, ensuring that only one task can execute on an actor at a time. This helps prevent data races and makes concurrent programming safer.
For more detailed information, you can refer to the session Explore Swift performance (24:33).
Relevant Sessions
- Explore Swift performance
- A Swift Tour: Explore Swift’s features and design
- Migrate your app to Swift 6
These sessions provide a comprehensive overview of concurrency in Swift, including the cooperative thread pool, async functions, and actors.
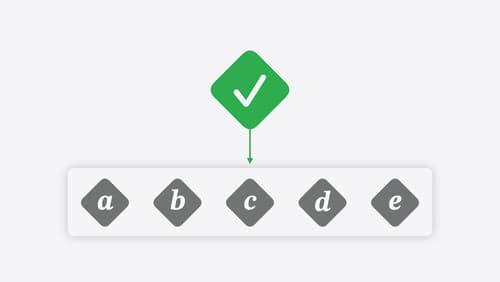
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
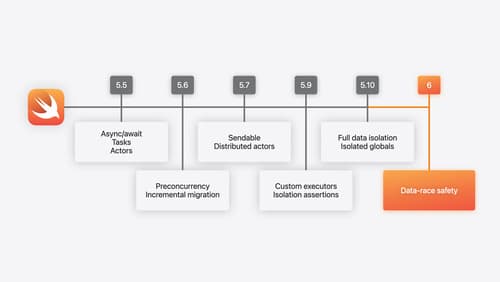
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
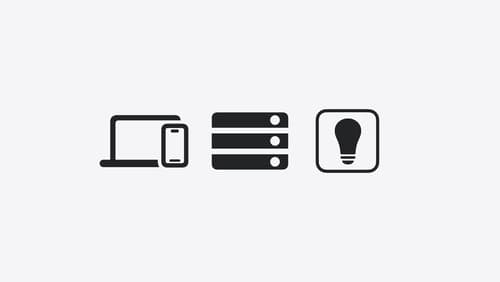
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
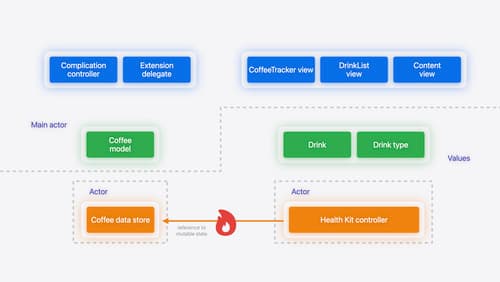
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
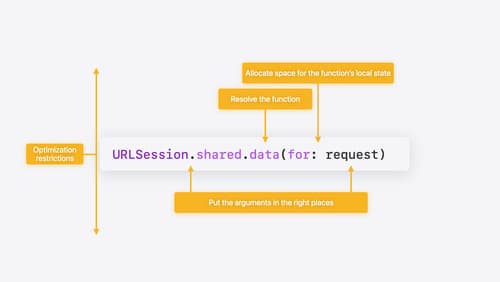
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.