Explain uncopyable types in detail
Asked on 08/08/2024
1 search
Noncopyable types in Swift are a new feature introduced to provide more control over value ownership and to prevent certain types of programming errors. Here’s a detailed explanation based on the context provided:
What are Noncopyable Types?
In Swift, most types are copyable by default, meaning that you can create multiple copies of a value. However, there are scenarios where copying a value can lead to errors or inefficiencies. Noncopyable types address these issues by suppressing the default copyability of a type.
How to Define Noncopyable Types
To define a noncopyable type, you use the ~copyable
keyword. This keyword suppresses the default conformance to the Copyable
protocol. For example:
struct FloppyDisk {
~copyable
// Type definition here
}
In this example, FloppyDisk
is a noncopyable type. Attempting to copy an instance of FloppyDisk
will result in a compile-time error.
Use Cases for Noncopyable Types
-
Unique Ownership: Noncopyable types are useful for representing resources that should have a single owner, such as file handles or network connections. This ensures that the resource is properly managed and prevents issues like multiple writes to the same file.
-
Performance Optimization: In low-level systems with severe resource constraints, copying values can be costly. Noncopyable types help in such scenarios by preventing unnecessary copies, thus optimizing performance.
-
Correctness: By preventing copies, noncopyable types can help avoid logical errors in your code. For example, in a banking application, you might want to ensure that a bank transfer cannot be accidentally executed more than once.
Example: Bank Transfer
Consider a type modeling a bank transfer:
struct BankTransfer {
~copyable
var state: TransferState
func run() {
// Complete the transfer
}
}
In this example, BankTransfer
is noncopyable, ensuring that each transfer is unique and cannot be accidentally duplicated.
Generics and Noncopyable Types
Swift 6 introduces support for noncopyable generics, allowing you to use noncopyable types in generic code. This is done by removing the Copyable
constraint from generic parameters:
func execute<T: Runnable>(task: T) {
// Execute the task
}
In this example, T
can be a noncopyable type, allowing for more flexible and safe generic programming.
Further Learning
To dive deeper into noncopyable types, you can watch the session Consume noncopyable types in Swift starting at the "Noncopyable Types" chapter.
Relevant Sessions
These sessions provide a comprehensive overview of noncopyable types, their use cases, and how to implement them in Swift.
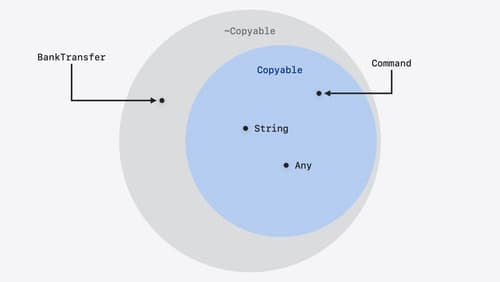
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.
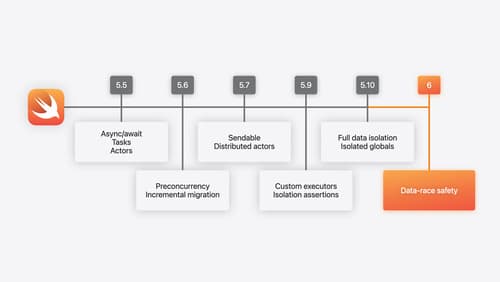
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.