how to use animation(_:body:)
Generated on 8/8/2024
1 search
To use the animation(_:body:)
function in SwiftUI, you can wrap your state changes within this function to apply animations to the resulting view updates. Here's a basic example:
import SwiftUI
struct ContentView: View {
@State private var isAnimating = false
var body: some View {
VStack {
Rectangle()
.fill(isAnimating ? Color.blue : Color.red)
.frame(width: 100, height: 100)
.animation(.easeInOut, value: isAnimating)
Button("Animate") {
withAnimation {
isAnimating.toggle()
}
}
}
}
}
In this example:
- The
Rectangle
changes its color based on theisAnimating
state. - The
animation(_:value:)
modifier is used to apply aneaseInOut
animation to the color change. - The
Button
toggles theisAnimating
state, and thewithAnimation
block ensures that the state change is animated.
For more detailed information on using animations in SwiftUI, you can refer to the session Enhance your UI animations and transitions (09:29). This session covers how to animate UIKit and AppKit views using SwiftUI animations and provides examples of bridging animations across different frameworks.
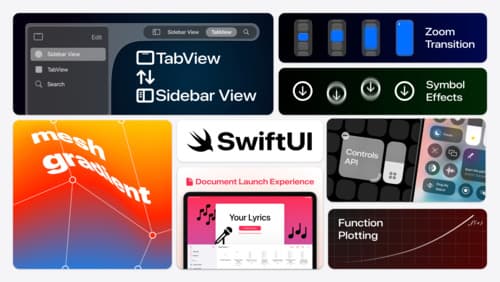
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
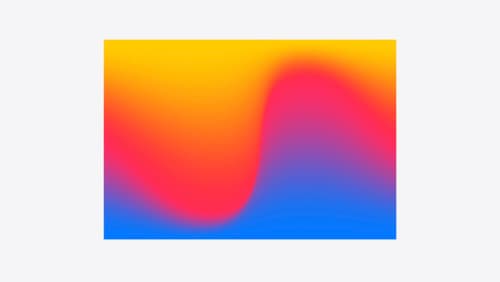
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
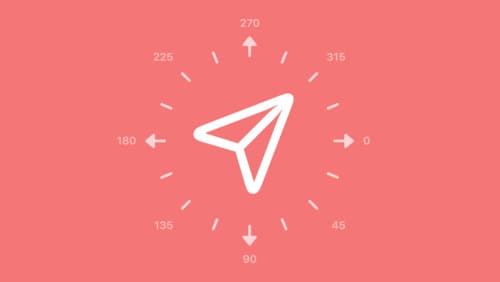
What’s new in SF Symbols 6
Explore the latest updates to SF Symbols, Apple’s library of iconography designed to integrate seamlessly with San Francisco, the system font for all Apple platforms. Learn how the new Wiggle, Rotate, and Breathe animation presets can bring vitality to your interface. To get the most out of this session, we recommend first watching “What’s new in SF Symbols 5” from WWDC23.
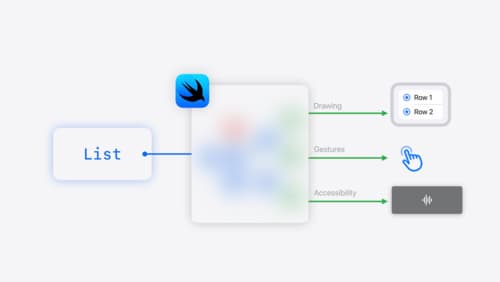
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
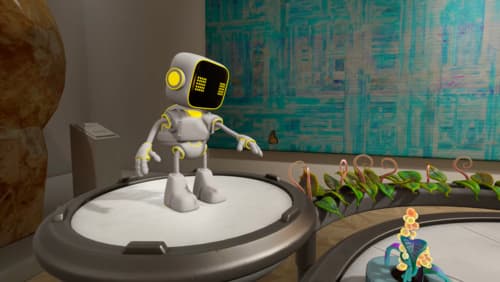
Compose interactive 3D content in Reality Composer Pro
Discover how the Timeline view in Reality Composer Pro can bring your 3D content to life. Learn how to create an animated story in which characters and objects interact with each other and the world around them using inverse kinematics, blend shapes, and skeletal poses. We’ll also show you how to use built-in and custom actions, sequence your actions, apply triggers, and implement natural movements.
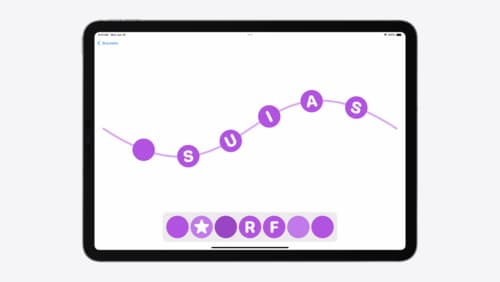
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
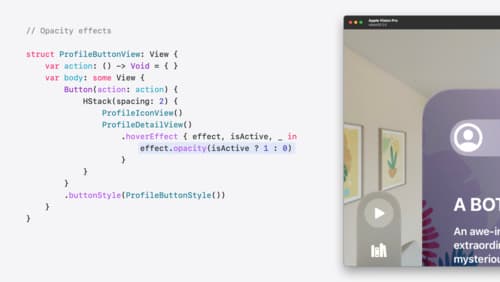
Create custom hover effects in visionOS
Learn how to develop custom hover effects that update views when people look at them. Find out how to build an expanding button effect that combines opacity, scale, and clip effects. Discover best practices for creating effects that are comfortable and respect people’s accessibility needs.