explain sendable
Generated on 8/8/2024
1 search
In Swift, the concept of "sendable" is crucial for ensuring data-race safety in concurrent programming. A type is considered sendable if it can be safely transferred between different concurrency domains, such as actors or threads, without causing data races. Here are some key points about sendable from the WWDC sessions:
-
Explicit Sendable Conformance:
- For internal types, Swift can automatically infer sendability. However, for public types, you need to explicitly mark them as sendable. This is because marking a type as sendable is a guarantee to your clients that the type contains no mutable state (Migrate your app to Swift 6).
-
Data-Race Safety:
- In Swift 6, the data-race safety of your program is fully verified at compile time. This is achieved by requiring that values shared between concurrency domains are sendable. A sendable value protects its state from concurrent accesses (A Swift Tour: Explore Swift’s features and design).
-
Practical Example:
- If you have a type that is a struct with immutable properties, it can be made sendable. This allows you to store the type in one actor and send it to another actor without risking data races (Migrate your app to Swift 6).
-
Handling Non-Sendable Types:
- If you encounter a type that cannot be made sendable, such as an Objective-C type that stores mutable state, you may need to make choices about safety. This could involve reasoning about the type's usage or adding synchronization manually (Migrate your app to Swift 6).
-
Actors and Sendable:
- Actors in Swift are reference types that can encapsulate shared mutable state. They help in making types sendable by ensuring that the state is accessed in a thread-safe manner (A Swift Tour: Explore Swift’s features and design).
By understanding and utilizing the sendable protocol, you can ensure that your Swift code is safe and free from data races when dealing with concurrency.
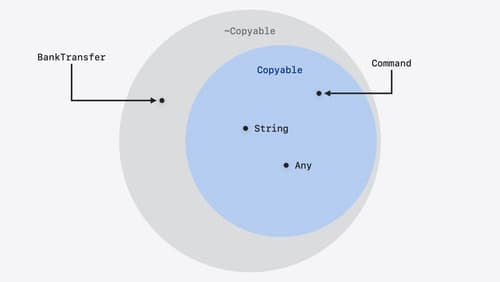
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.
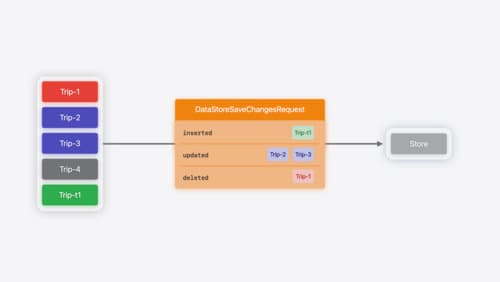
Create a custom data store with SwiftData
Combine the power of SwiftData’s expressive, declarative modeling API with your own persistence backend. Learn how to build a custom data store and explore how to progressively add persistence features in your app. To get the most out of this session, watch “Meet SwiftData” and “Model your schema with SwiftData” from WWDC23.
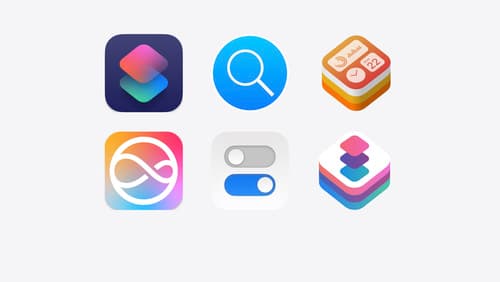
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.
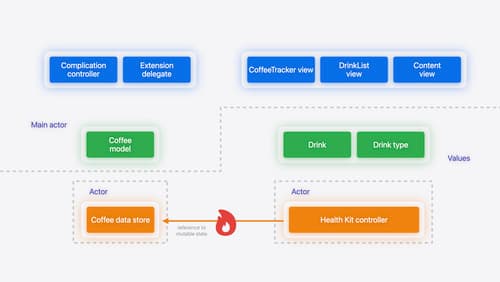
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
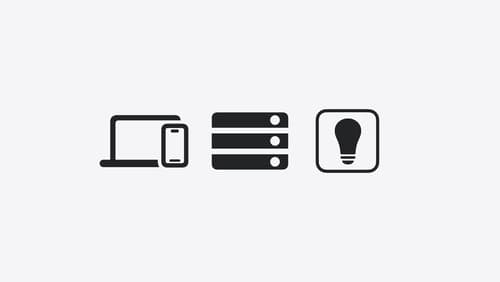
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.