how do I implement certificate pinning
Generated on 8/8/2024
1 search
To implement certificate pinning, you would typically follow these steps:
-
Obtain the Certificate: Get the public key or the certificate of the server you want to pin. This can be done by connecting to the server and extracting the certificate.
-
Embed the Certificate: Embed the certificate or its public key in your application. This can be done by adding it to your app's resources.
-
Validate the Certificate: During the SSL/TLS handshake, validate the server's certificate against the pinned certificate. If the server's certificate does not match the pinned certificate, the connection should be terminated.
Here is a basic example in Swift for iOS:
import Foundation
class PinnedURLSessionDelegate: NSObject, URLSessionDelegate {
func urlSession(_ session: URLSession, didReceive challenge: URLAuthenticationChallenge, completionHandler: @escaping (URLSession.AuthChallengeDisposition, URLCredential?) -> Void) {
if let serverTrust = challenge.protectionSpace.serverTrust,
SecTrustEvaluateWithError(serverTrust, nil) {
let serverCertificate = SecTrustGetCertificateAtIndex(serverTrust, 0)
let serverCertificateData = SecCertificateCopyData(serverCertificate!) as Data
let localCertificateData = NSData(contentsOfFile: Bundle.main.path(forResource: "your_certificate", ofType: "cer")!)! as Data
if serverCertificateData == localCertificateData {
completionHandler(.useCredential, URLCredential(trust: serverTrust))
return
}
}
completionHandler(.cancelAuthenticationChallenge, nil)
}
}
In this example:
your_certificate.cer
is the certificate file you have pinned.- The
PinnedURLSessionDelegate
class handles the URL session's authentication challenge by comparing the server's certificate with the pinned certificate.
For more detailed information on implementing security features like certificate pinning, you might want to check out sessions related to security and privacy from WWDC. For instance, the session What’s new in privacy covers various privacy and security enhancements, which might provide additional context and best practices.
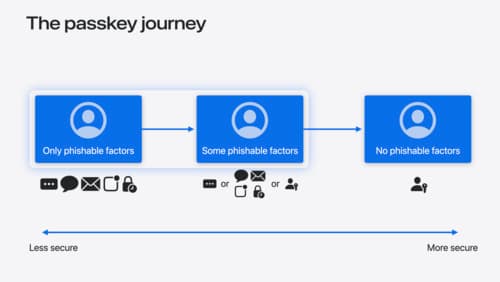
Streamline sign-in with passkey upgrades and credential managers
Learn how to automatically upgrade existing, password-based accounts to use passkeys. We’ll share why and how to improve account security and ease of sign-in, information about new features available for credential manager apps, and how to make your app information shine in the new Passwords app.
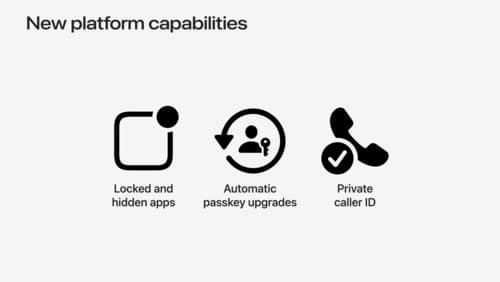
What’s new in privacy
At Apple, we believe privacy is a fundamental human right. Learn about new and improved permission flows and other features that manage data in a privacy-preserving way, so that you can focus on creating great app experiences.