How create av recorder for audio
Generated on 8/8/2024
1 search
To create an AV recorder for audio, you can use the AVFoundation framework. Here’s a basic outline of how you can set up an AV recorder for audio:
-
Create an AV capture session: This session coordinates the data flow between the input (microphone) and the output (file).
-
Set up the audio input: Use an
AVCaptureDevice
to represent the microphone. -
Set up the audio output: Use an
AVCaptureAudioDataOutput
to manage the output and write it to disk. -
Connect the input and output: Use an
AVCaptureConnection
to connect the input and output. -
Start the session: Begin the capture session to start recording.
Here’s a sample code snippet to illustrate these steps:
import AVFoundation
// Create a capture session
let captureSession = AVCaptureSession()
// Set up the audio input
guard let audioDevice = AVCaptureDevice.default(for: .audio) else {
fatalError("No audio device found")
}
let audioInput = try AVCaptureDeviceInput(device: audioDevice)
captureSession.addInput(audioInput)
// Set up the audio output
let audioOutput = AVCaptureAudioDataOutput()
captureSession.addOutput(audioOutput)
// Start the session
captureSession.startRunning()
// Set up a file output
let outputFileURL = URL(fileURLWithPath: "path/to/save/audio/file.m4a")
let fileOutput = AVCaptureMovieFileOutput()
captureSession.addOutput(fileOutput)
// Start recording
fileOutput.startRecording(to: outputFileURL, recordingDelegate: self)
For more detailed information, you can refer to the session Build compelling spatial photo and video experiences from WWDC 2024, which covers setting up AV capture sessions and managing inputs and outputs.
If you are interested in capturing screen audio and microphone content specifically, you can use the new API provided by ScreenCaptureKit. For more details, refer to the session Capture HDR content with ScreenCaptureKit.
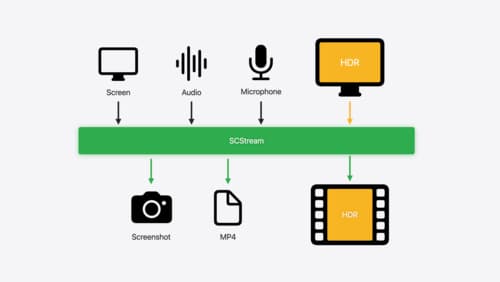
Capture HDR content with ScreenCaptureKit
Learn how to capture high dynamic colors using ScreenCaptureKit, and explore new features like HDR support, microphone capture, and straight-to-file recording.
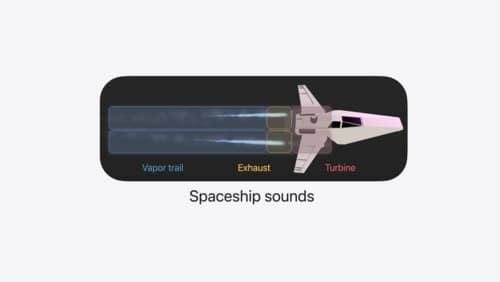
Enhance your spatial computing app with RealityKit audio
Elevate your spatial computing experience using RealityKit audio. Discover how spatial audio can make your 3D immersive experiences come to life. From ambient audio, reverb, to real-time procedural audio that can add character to your 3D content, learn how RealityKit audio APIs can help make your app more engaging.
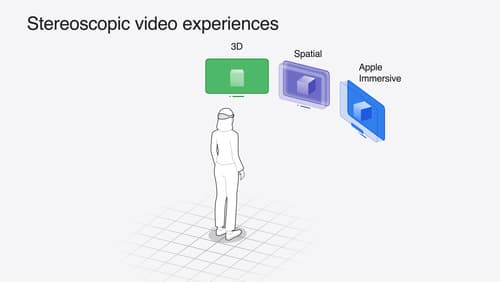
Build compelling spatial photo and video experiences
Learn how to adopt spatial photos and videos in your apps. Explore the different types of stereoscopic media and find out how to capture spatial videos in your iOS app on iPhone 15 Pro. Discover the various ways to detect and present spatial media, including the new QuickLook Preview Application API in visionOS. And take a deep dive into the metadata and stereo concepts that make a photo or video spatial.