how can I set the app icon badge
Generated on 8/9/2024
1 search
To set the app icon badge, you can use the UNUserNotificationCenter
to manage notifications and update the badge count. Here's a basic example of how you can achieve this:
-
Request Notification Permissions: First, you need to request permission to display notifications.
import UserNotifications UNUserNotificationCenter.current().requestAuthorization(options: [.badge, .sound, .alert]) { (granted, error) in if granted { print("Permission granted") } else if let error = error { print("Error: \(error.localizedDescription)") } }
-
Update the Badge Count: You can update the badge count by setting the
badge
property of aUNMutableNotificationContent
object.let content = UNMutableNotificationContent() content.badge = NSNumber(value: 1) // Set the badge number let trigger = UNTimeIntervalNotificationTrigger(timeInterval: 1, repeats: false) let request = UNNotificationRequest(identifier: "badgeNotification", content: content, trigger: trigger) UNUserNotificationCenter.current().add(request) { (error) in if let error = error { print("Error: \(error.localizedDescription)") } }
-
Clear the Badge Count: To clear the badge count, you can set it to
0
.UIApplication.shared.applicationIconBadgeNumber = 0
For more detailed information on how to extend your app's controls across the system, you can refer to the session Extend your app’s controls across the system (12:25).
If you need more information on how to integrate these features into your app, you might find the session Bring your app’s core features to users with App Intents (08:06) helpful.
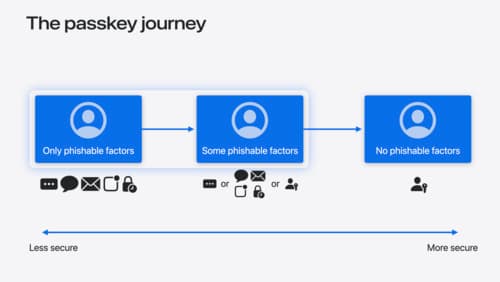
Streamline sign-in with passkey upgrades and credential managers
Learn how to automatically upgrade existing, password-based accounts to use passkeys. We’ll share why and how to improve account security and ease of sign-in, information about new features available for credential manager apps, and how to make your app information shine in the new Passwords app.
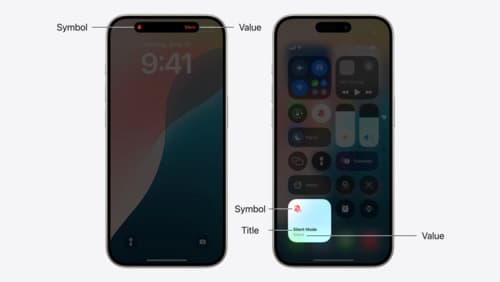
Extend your app’s controls across the system
Bring your app’s controls to Control Center, the Lock Screen, and beyond. Learn how you can use WidgetKit to extend your app’s controls to the system experience. We’ll cover how you can to build a control, tailor its appearance, and make it configurable.
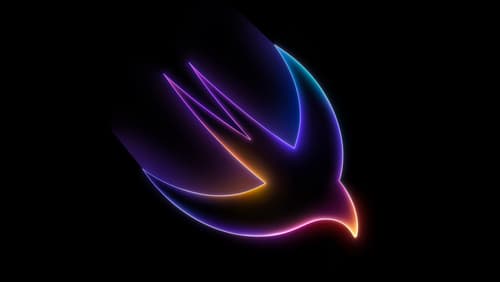
Platforms State of the Union
Discover the newest advancements on Apple platforms.
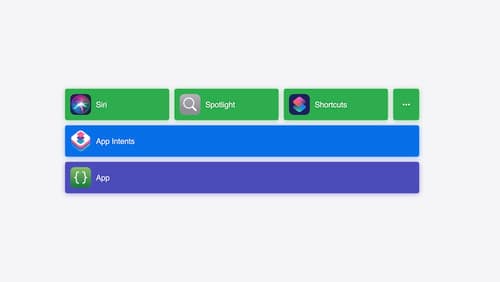
Bring your app’s core features to users with App Intents
Learn the principles of the App Intents framework, like intents, entities, and queries, and how you can harness them to expose your app’s most important functionality right where people need it most. Find out how to build deep integration between your app and the many system features built on top of App Intents, including Siri, controls and widgets, Apple Pencil, Shortcuts, the Action button, and more. Get tips on how to build your App Intents integrations efficiently to create the best experiences in every surface while still sharing code and core functionality.
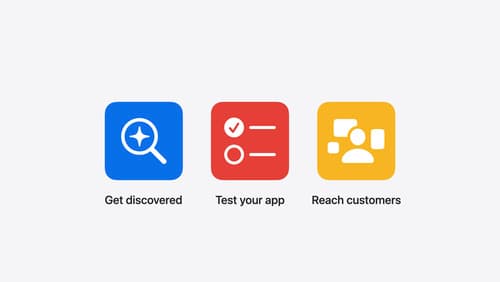
What’s new in App Store Connect
Explore new features for discovery, testing, and marketing. Find out how to nominate your apps for featuring on the App Store, share exciting moments (like a version launch) with marketing assets generated for you, deep link to specific content in your app from custom product pages, use the latest enhancements to TestFlight, and more.