How to create scroll view?
Generated on 8/9/2024
1 search
To create a scroll view in SwiftUI, you can use the ScrollView
component. Here are some examples and techniques mentioned in the WWDC sessions:
-
Basic Scroll View:
- You can create a simple horizontal or vertical scroll view by wrapping your content in a
ScrollView
and specifying the axis of scrolling.
ScrollView(.vertical) { VStack { ForEach(0..<50) { index in Text("Item \(index)") .padding() } } }
- You can create a simple horizontal or vertical scroll view by wrapping your content in a
-
Custom Scroll Effects:
- You can use the
scrollTransition
modifier to create custom scroll effects. This allows you to change the appearance of elements based on their position in the scroll view.
ScrollView(.horizontal) { HStack { ForEach(0..<10) { index in Image(systemName: "photo") .resizable() .frame(width: 100, height: 100) .scrollTransition { content, phase in content .rotationEffect(.degrees(phase.value * 360)) } } } }
For more details on creating custom scroll effects, you can refer to the session Create custom visual effects with SwiftUI (00:02:07).
- You can use the
-
Advanced Scroll View Modifiers:
- In tvOS 18, new view modifiers specific to
ScrollView
have been introduced. These include modifiers to handle visibility changes and scroll target behavior.
ScrollView { VStack { // Your content here } .onScrollVisibilityChange { isVisible in // Handle visibility change } .scrollTargetBehavior(.viewAligned) }
For more information on these new modifiers, you can check the session Migrate your TVML app to SwiftUI (00:10:07).
- In tvOS 18, new view modifiers specific to
-
Programmatic Control and Enhancements:
- SwiftUI provides more programmatic control over scroll views, including the ability to scroll to specific positions and control content alignment.
ScrollView { VStack { // Your content here } .scrollPosition(.top) .scrollBounceBehavior(.disabled) }
For more details on these enhancements, refer to the session What’s new in SwiftUI (00:16:18).
By using these techniques, you can create and customize scroll views in SwiftUI to fit your app's needs.
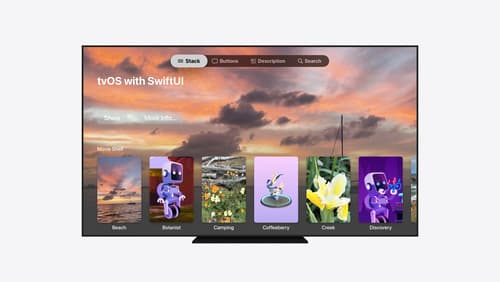
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
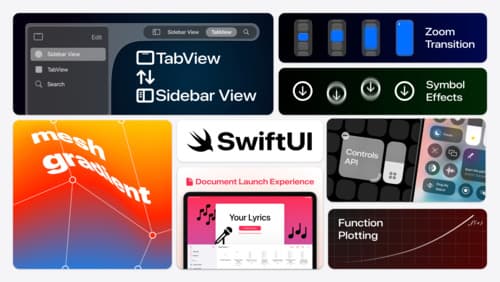
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
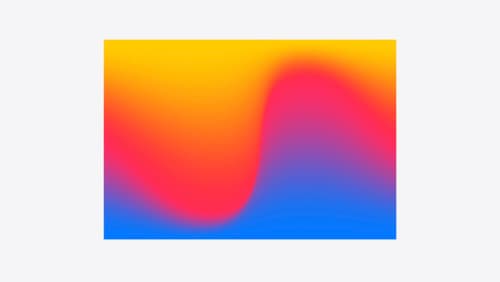
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.