teach me everything about memory management
Generated on 8/9/2024
1 search
To understand memory management, especially in the context of Apple's WWDC sessions, we can break it down into several key areas:
Heap Memory
Heap memory is a critical part of your app's memory management. It is used for dynamic memory allocation, where memory is allocated and freed at runtime. Here are some important points about heap memory:
-
Heap Memory Overview:
- Heap memory is used for long-lived memory allocations.
- It is made up of multiple virtual memory regions, each broken into 16 KB memory pages.
- Pages can be clean, dirty, or swapped. Clean pages are cheap as they can be discarded and reloaded from disk, while dirty pages count towards the app's memory footprint (Analyze heap memory).
-
Memory Allocation:
- Memory allocation in the heap is flexible but more expensive compared to stack or global memory.
- Swift uses the heap for class instances and other features requiring shared ownership (Explore Swift performance).
-
Tools for Inspecting Heap Memory:
- Xcode provides tools to measure and inspect heap memory usage.
- Malloc stack logging can be enabled in Xcode to track where and when memory was allocated (Analyze heap memory).
Memory Leaks
Memory leaks occur when memory that is no longer needed is not released. This can lead to increased memory usage and potential app crashes.
-
Types of Memory:
- Useful memory: Reachable and will be used again.
- Abandoned memory: Reachable but won't be used again.
- Leaked memory: Unreachable and can't be used again (Analyze heap memory).
-
Fixing Memory Leaks:
- Identify and fix reference cycles or lost pointers.
- Use tools like the Xcode memory graph debugger to find and resolve leaks (Analyze heap memory).
Managing Memory Growth
Memory growth can be transient or persistent, and managing it is crucial for app performance.
-
Transient Memory Growth:
- Often caused by temporary allocations that are not immediately freed.
- Auto release pools in Objective-C and Swift can lead to temporary memory growth (Analyze heap memory).
-
Persistent Memory Growth:
- Caused by long-lived allocations that are not released.
- Requires careful tracking and management to ensure memory is freed when no longer needed (Analyze heap memory).
Performance Considerations
Memory management impacts app performance significantly. Efficient memory allocation and deallocation can improve app responsiveness and reduce crashes.
-
Low-Level Principles:
- Different types of memory (global, stack, heap) have different performance characteristics.
- Heap memory is flexible but more expensive to manage (Explore Swift performance).
-
Improving Runtime Performance:
- Reducing memory footprint and managing memory efficiently can lead to better app performance.
- Use tools and best practices to optimize memory usage (Analyze heap memory).
Sessions for Further Learning
These sessions provide a comprehensive overview of memory management, tools for inspecting memory usage, and strategies for optimizing memory in your applications.
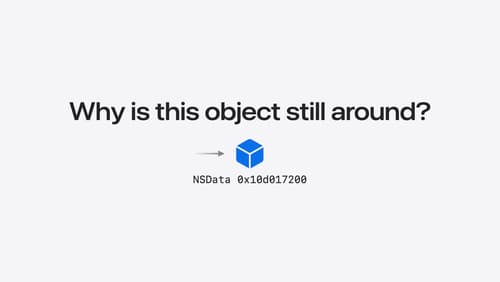
Analyze heap memory
Dive into the basis for your app’s dynamic memory: the heap! Explore how to use Instruments and Xcode to measure, analyze, and fix common heap issues. We’ll also cover some techniques and best practices for diagnosing transient growth, persistent growth, and leaks in your app.
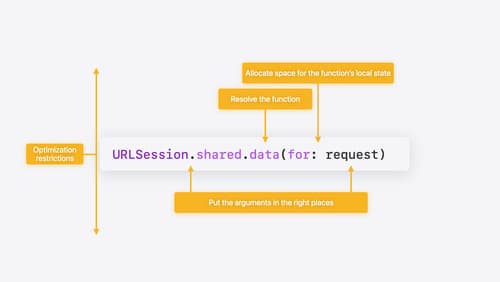
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.