How to handle navigation in SwiftUI?
Asked on 08/09/2024
1 search
To handle navigation in SwiftUI, you can utilize the NavigationView
and NavigationLink
components. SwiftUI provides a declarative way to manage navigation, which simplifies the process of creating complex navigation flows.
Key Concepts:
-
NavigationView: This is the container that manages the navigation stack. It is used to wrap the views that you want to navigate between.
-
NavigationLink: This is used to create a link to another view. When tapped, it pushes the destination view onto the navigation stack.
-
State Management: SwiftUI's state-driven approach ensures that your UI stays in sync with your data. When the state changes, SwiftUI automatically updates the UI.
-
Transitions: SwiftUI allows you to customize transitions between views. For example, you can use a zoom transition to create a more interactive experience. This can be done by using the
navigationTransitionStyle
modifier to specify the transition style.
Example:
Here's a simple example of how you might set up navigation in SwiftUI:
import SwiftUI
struct ContentView: View {
var body: some View {
NavigationView {
VStack {
NavigationLink(destination: DetailView()) {
Text("Go to Detail View")
}
}
.navigationTitle("Home")
}
}
}
struct DetailView: View {
var body: some View {
Text("This is the detail view")
.navigationTitle("Detail")
}
}
Advanced Techniques:
-
Custom Transitions: You can enhance your navigation with custom transitions. For example, using a zoom transition can be achieved by adding the
navigationTransitionStyle
modifier to the presented view and specifying the zoom transition. This is discussed in the Enhance your UI animations and transitions session. -
State-Driven Updates: SwiftUI automatically updates the UI when the state changes, which is a core principle of its design. This is covered in the SwiftUI essentials session.
For more detailed information, you can refer to the sessions mentioned above, which provide insights into handling navigation and transitions in SwiftUI.
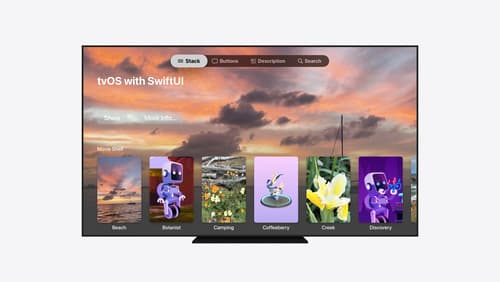
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
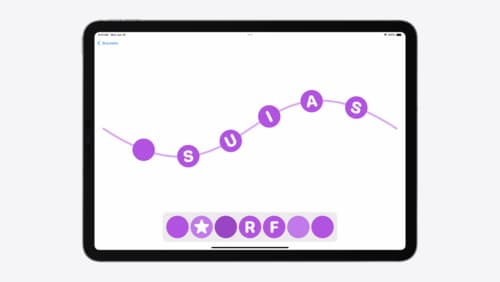
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
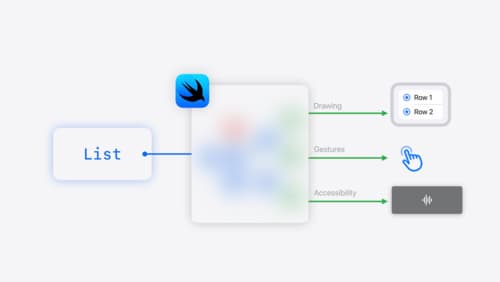
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.