How to make a navigation with SwiftUi
Generated on 8/10/2024
1 search
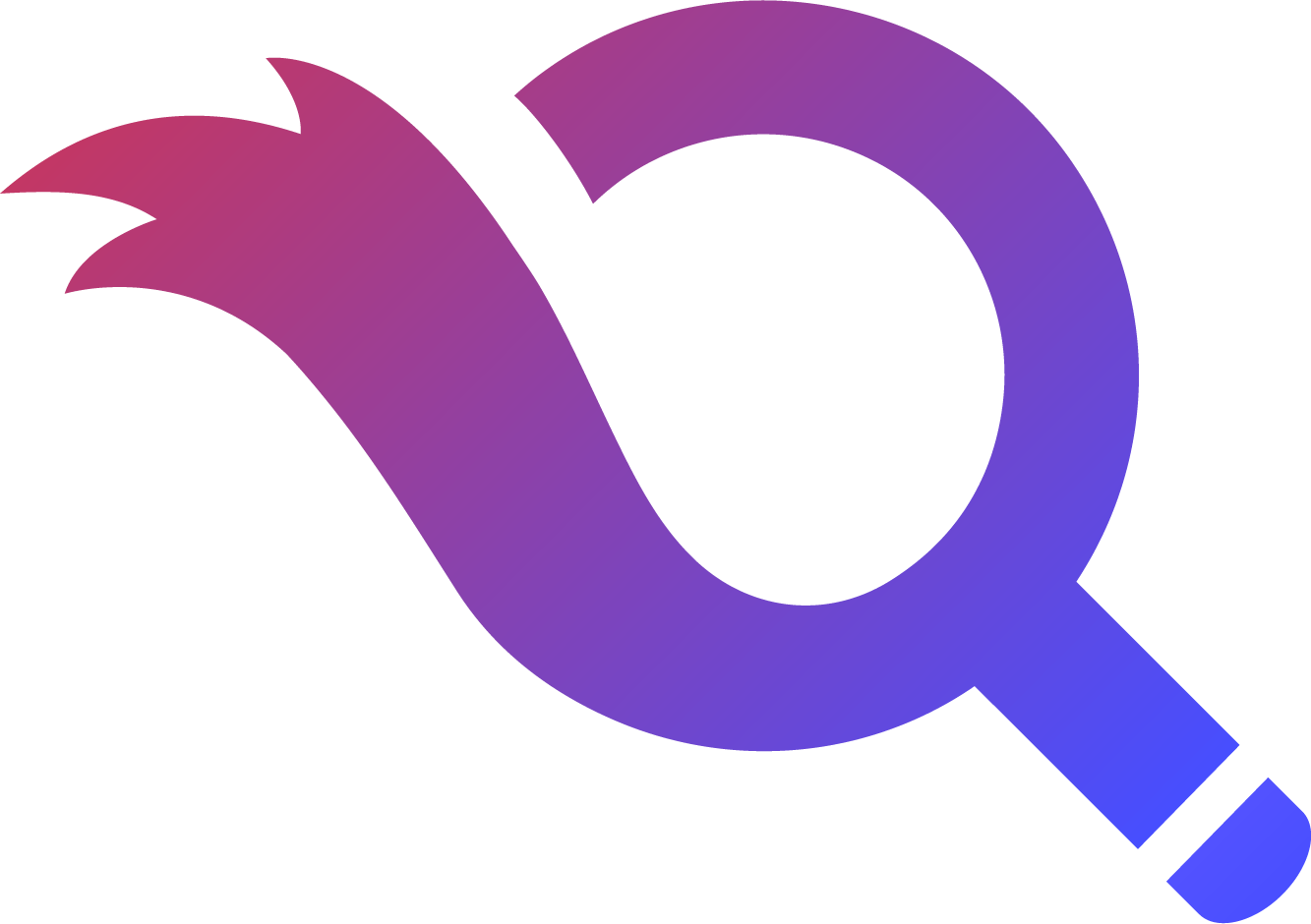
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To create navigation in SwiftUI, you can use the NavigationView
and NavigationLink
components. These components allow you to build a navigation hierarchy in your app. Here's a basic example:
import SwiftUI
struct ContentView: View {
var body: some View {
NavigationView {
List {
NavigationLink(destination: DetailView()) {
Text("Go to Detail View")
}
}
.navigationTitle("Main View")
}
}
}
struct DetailView: View {
var body: some View {
Text("This is the Detail View")
.navigationTitle("Detail View")
}
}
In this example, NavigationView
is used to wrap the content that should be navigable. NavigationLink
is used to define a link to another view, in this case, DetailView
. When the user taps on the text "Go to Detail View," they are navigated to the DetailView
.
For more advanced navigation, you can explore the navigationSplitView
for creating split views or customizable multicolumn tables, as mentioned in the SwiftUI essentials session.
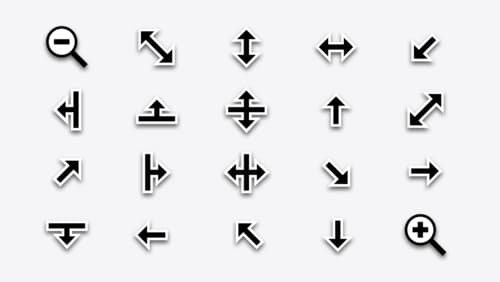
What’s new in AppKit
Discover the latest advances in Mac app development. Get an overview of the new features in macOS Sequoia, and how to adopt them in your app. Explore new ways to integrate your existing code with SwiftUI. Learn about the improvements made to numerous AppKit controls, like toolbars, menus, text input, and more.
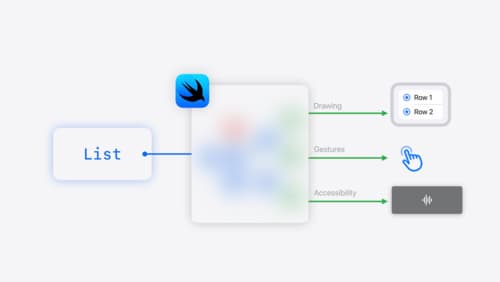
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
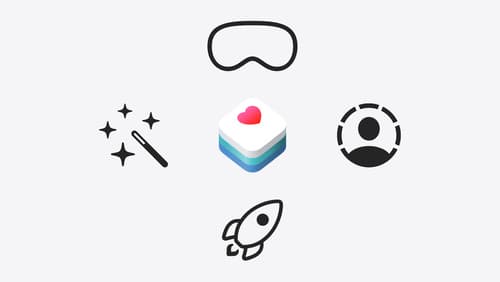
Get started with HealthKit in visionOS
Discover how to use HealthKit to create experiences that take full advantage of the spatial canvas. Learn the capabilities of HealthKit on the platform, find out how to bring an existing iPadOS app to visionOS, and explore the special considerations governing HealthKit during a Guest User session. You’ll also learn ways to use SwiftUI, Swift Charts, and Swift concurrency to craft innovative experiences with HealthKit.
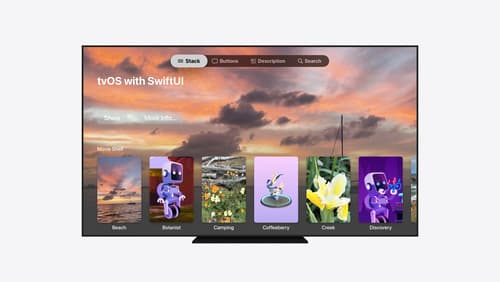
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
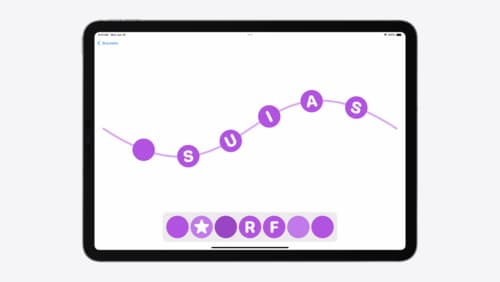
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.