how to use async await
Generated on 8/12/2024
1 search
To use async
and await
in Swift, you need to understand how they work to handle asynchronous operations. Here's a brief overview based on the content from WWDC:
-
Async Functions: In Swift, a function that can suspend its execution to wait for an asynchronous operation is marked with the
async
keyword. This indicates that the function can perform asynchronous tasks. -
Await Keyword: When calling an
async
function, you use theawait
keyword to indicate that the function may suspend at that point. This allows other tasks to execute while waiting for the asynchronous operation to complete. -
Concurrency in Swift: Swift's concurrency model allows you to write code that can perform multiple tasks simultaneously. This is particularly useful for operations like reading from disk or making network requests, where you don't want to block the main thread.
-
Actors: Swift introduces actors, which are reference types that protect their state by serializing access. Calls to actor methods from outside the actor's context are asynchronous, and you need to use
await
to access them.
For a practical example, you can refer to the session A Swift Tour: Explore Swift’s features and design (19:10) where the use of async
and await
is demonstrated in a server development environment.
Additionally, the session Explore Swift performance (24:33) discusses how async functions are implemented and how they manage memory allocation differently from synchronous functions.
These sessions provide a comprehensive look at how Swift handles asynchronous operations and concurrency, making it easier to write efficient and safe concurrent code.
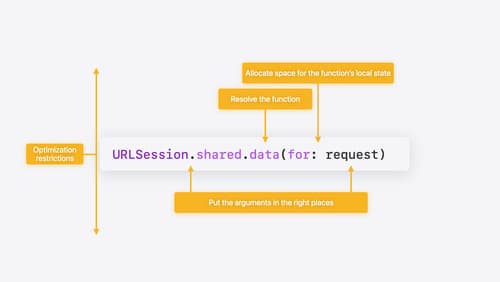
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
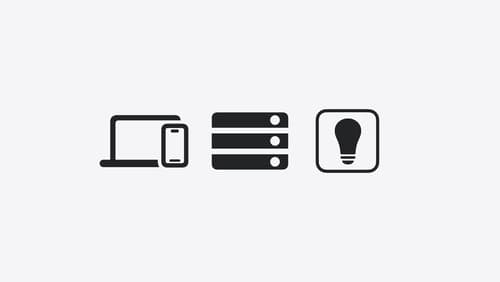
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
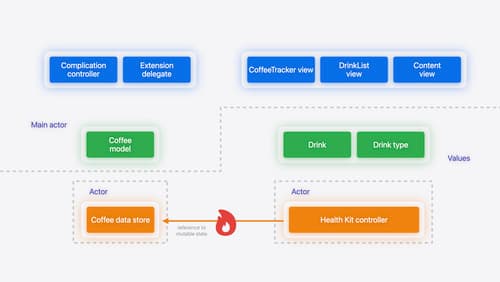
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
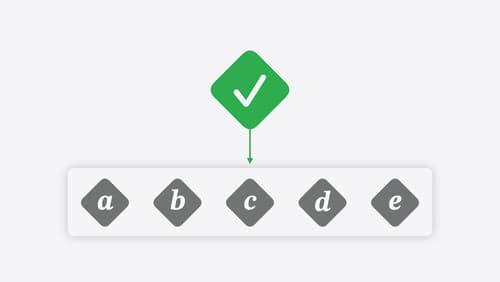
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.