Markdown to SwiftUI text
Generated on 8/12/2024
1 search
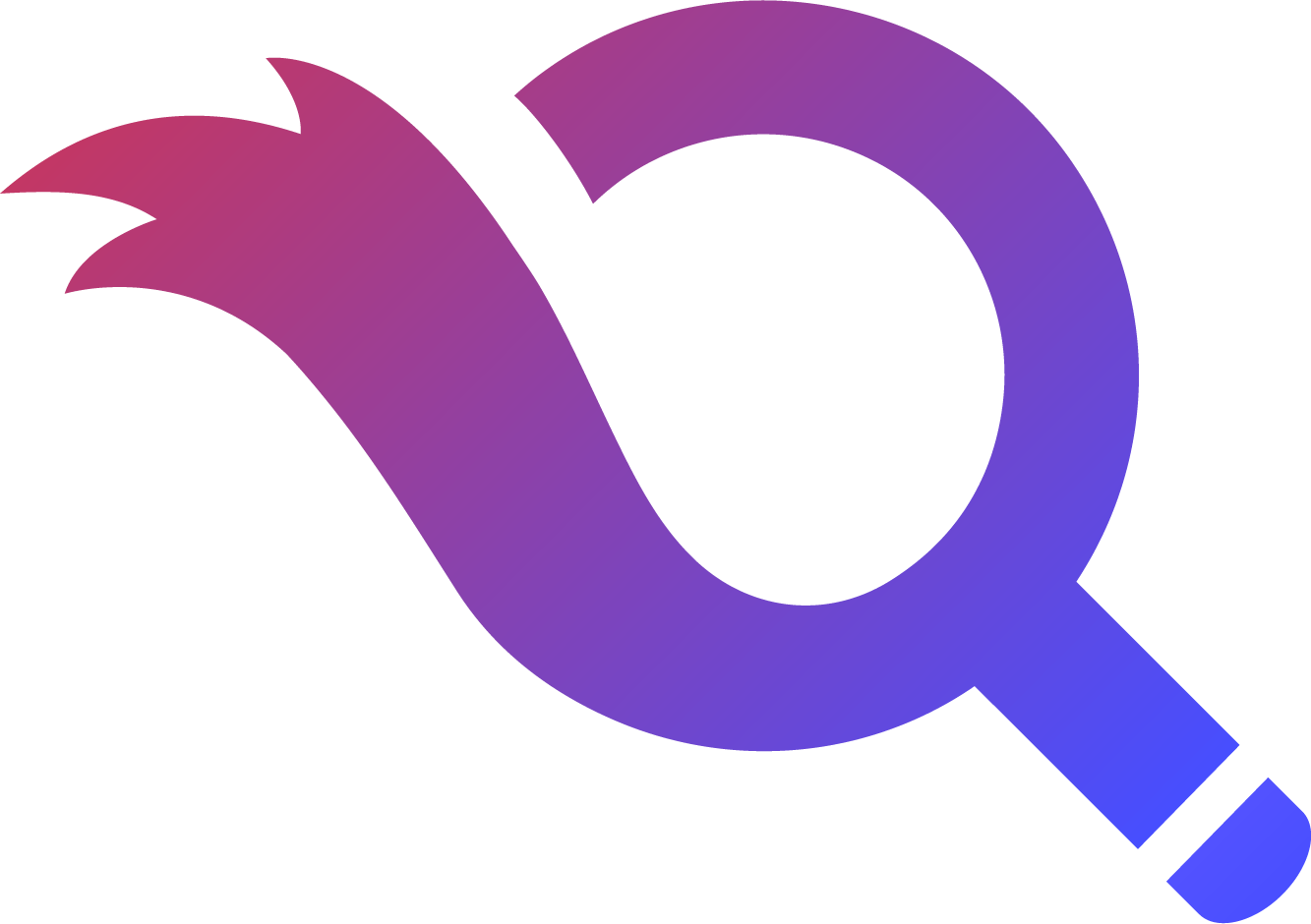
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To convert Markdown to SwiftUI text, you can use SwiftUI's Text
view to display text content. SwiftUI does not directly support Markdown syntax, but you can manually format the text using SwiftUI's text modifiers. Here's a basic example of how you might convert Markdown to SwiftUI text:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack(alignment: .leading) {
Text("**Bold Text**")
.fontWeight(.bold)
Text("_Italic Text_")
.italic()
Text("[Link](https://www.example.com)")
.underline()
.foregroundColor(.blue)
}
.padding()
}
}
In this example, Markdown elements like bold and italic are manually styled using SwiftUI's text modifiers such as .fontWeight(.bold)
and .italic()
. Links can be styled with .underline()
and a color to simulate a hyperlink appearance.
For more advanced Markdown rendering, you might need to use a third-party library or custom parsing logic to interpret Markdown syntax and apply the appropriate SwiftUI text modifiers.
If you're interested in learning more about text handling in SwiftUI, you might find the session What's new in SwiftUI helpful, as it covers new ways to work with text and manage selection.
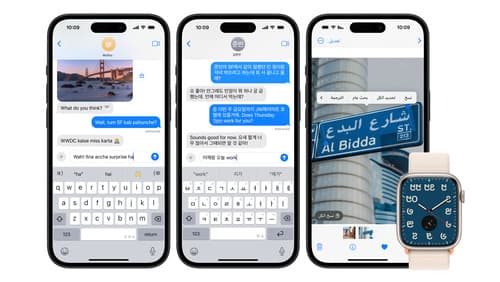
Build multilingual-ready apps
Ensure your app works properly and effectively for multilingual users. Learn best practices for text input, display, search, and formatting. Get details on typing in multiple languages without switching between keyboards. And find out how the latest advances in the String Catalog can make localization even easier.
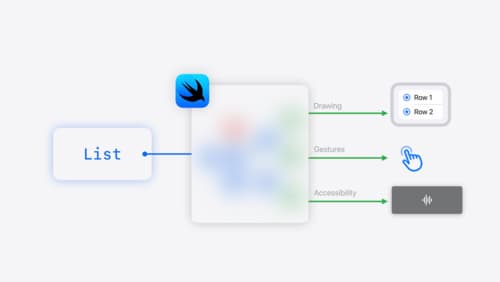
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
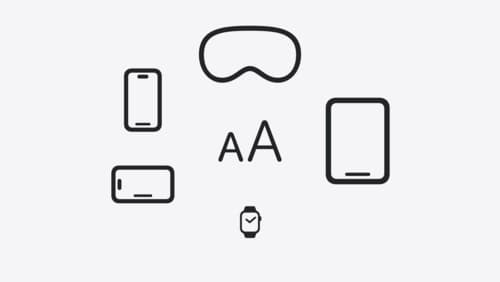
Get started with Dynamic Type
Dynamic Type lets people choose their preferred text size across the system and all of their apps. To help you get started supporting Dynamic Type, we’ll cover the fundamentals: How it works, how to find issues with scaling text in your app, and how to take practical steps using SwiftUI and UIKit to create a great Dynamic Type experience. We’ll also show how you can best use the Large Content Viewer to make navigation controls accessible to everyone.
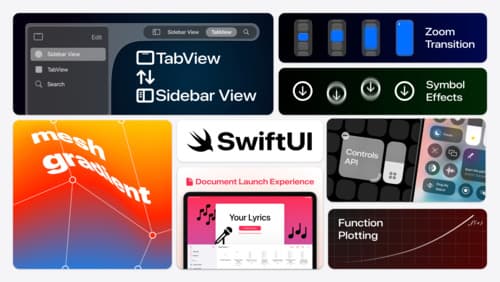
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
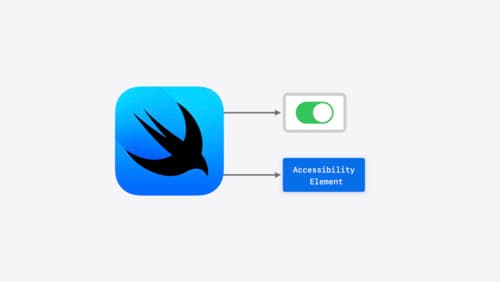
Catch up on accessibility in SwiftUI
SwiftUI makes it easy to build amazing experiences that are accessible to everyone. We’ll discover how assistive technologies understand and navigate your app through the rich accessibility elements provided by SwiftUI. We’ll also discuss how you can further customize these experiences by providing more information about your app’s content and interactions by using accessibility modifiers.