How to use a non sendable class with async methods correctly
Asked on 08/12/2024
1 search
To use a non-sendable class with async methods correctly in Swift, you can leverage Swift's concurrency features, particularly actors. Actors are a convenient way to manage shared mutable state safely across different concurrency domains. Here's a brief overview of how you can handle non-sendable classes with async methods:
-
Understanding Sendability: In Swift, a sendable value is one that can be safely shared across concurrency domains without causing data races. If a class is not sendable, it means it might not be safe to access it concurrently from multiple threads.
-
Using Actors: Actors in Swift are reference types that automatically protect their state by serializing access. This means that only one task can execute on an actor at a time, which helps prevent data races. If you have a non-sendable class, you can encapsulate it within an actor to manage its state safely.
-
Asynchronous Access: When you access an actor's methods from outside its context, these calls are asynchronous. You need to use the
await
keyword to handle these asynchronous calls properly.
For example, if you have a non-sendable class UserStore
, you can make it an actor to ensure safe concurrent access:
actor UserStore {
// Your shared mutable state and methods
}
// Usage
let userStore = UserStore()
await userStore.someMethod()
By making UserStore
an actor, you ensure that all accesses to its state are serialized, thus avoiding data races.
For more detailed information, you can refer to the session A Swift Tour: Explore Swift’s features and design (21:38) where actors and their usage are discussed.
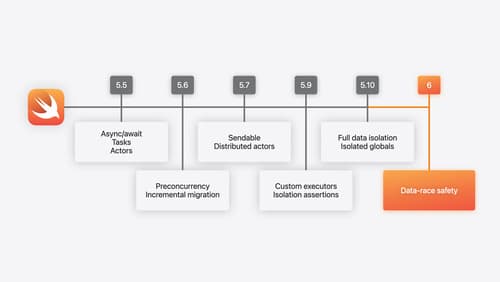
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
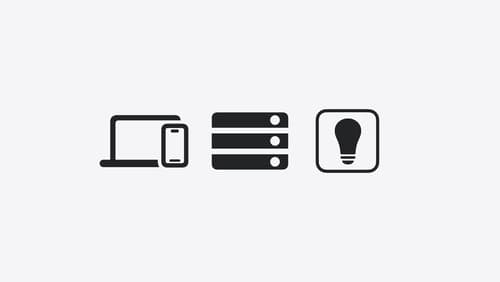
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
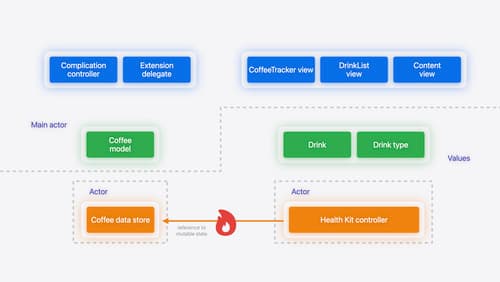
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.