class vs struct
Generated on 8/13/2024
1 search
In Swift, the choice between using a class or a struct depends on the semantics you want for your data type. Here's a summary based on the content from the WWDC sessions:
Structs
- Value Semantics: Structs are value types, meaning that when you copy a struct, you are copying all of its data. This is useful when you want each instance to be independent of others. For example, if you have a
Player
struct and you copy it, changes to the copy do not affect the original (Consume noncopyable types in Swift). - Inline Storage: Structs use inline storage, which means their data is stored directly in the memory location of the variable. This can be more efficient for small data types (Explore Swift performance).
- Mutability Control: You can control mutability using
let
andvar
. A struct declared withlet
is immutable (A Swift Tour: Explore Swift’s features and design).
Classes
- Reference Semantics: Classes are reference types, meaning that when you copy a class, you are copying a reference to the same instance. This is useful for shared mutable state (A Swift Tour: Explore Swift’s features and design).
- Out of Line Storage: Classes use out of line storage, which means their data is stored separately from the variable that holds the reference. This can be more efficient for large data types that are copied frequently (Explore Swift performance).
- Automatic Reference Counting: Swift uses automatic reference counting (ARC) to manage memory for class instances, which can lead to issues like retain cycles if not managed properly (A Swift Tour: Explore Swift’s features and design).
When to Use Each
- Use structs when you want value semantics, immutability, and when the data type is small and frequently copied.
- Use classes when you need shared mutable state, identity, or inheritance.
For more detailed insights, you can refer to the sessions:
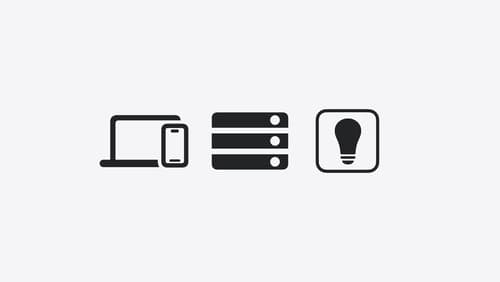
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
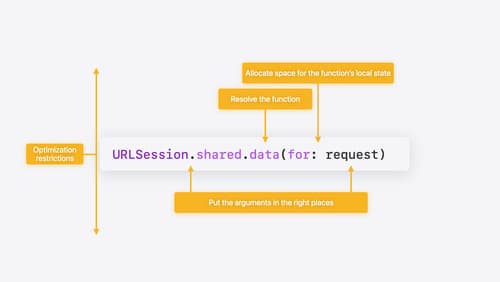
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
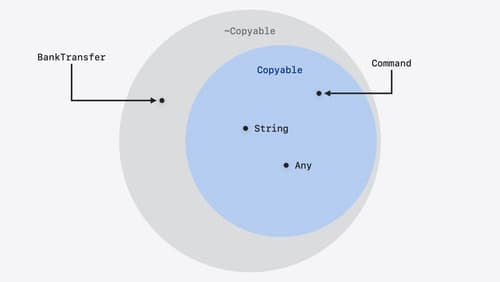
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.