What are the SwiftUI apis for making network calls?
Generated on 8/14/2024
1 search
SwiftUI itself does not provide specific APIs for making network calls. Instead, SwiftUI is designed to work seamlessly with other frameworks and technologies that handle networking. For making network calls in a SwiftUI app, you would typically use Swift's URLSession
or other networking libraries like Alamofire
. These can be integrated into your SwiftUI app to fetch data from the network and then update your SwiftUI views accordingly.
For example, you can use URLSession
to perform network requests and then use the data to update your SwiftUI views. Here's a simple example of how you might fetch data using URLSession
:
import SwiftUI
struct ContentView: View {
@State private var data: String = "Loading..."
var body: some View {
Text(data)
.onAppear {
fetchData()
}
}
func fetchData() {
guard let url = URL(string: "https://api.example.com/data") else { return }
URLSession.shared.dataTask(with: url) { data, response, error in
if let data = data, let string = String(data: data, encoding: .utf8) {
DispatchQueue.main.async {
self.data = string
}
}
}.resume()
}
}
This example demonstrates how to perform a network request and update a SwiftUI view with the fetched data. SwiftUI's declarative nature allows you to easily update the UI in response to changes in your data model.
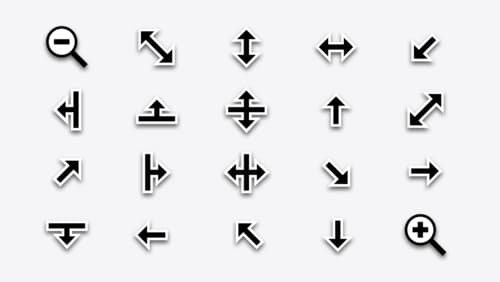
What’s new in AppKit
Discover the latest advances in Mac app development. Get an overview of the new features in macOS Sequoia, and how to adopt them in your app. Explore new ways to integrate your existing code with SwiftUI. Learn about the improvements made to numerous AppKit controls, like toolbars, menus, text input, and more.
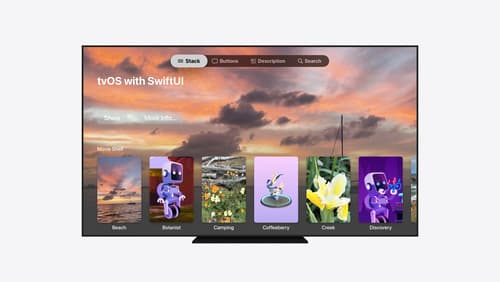
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
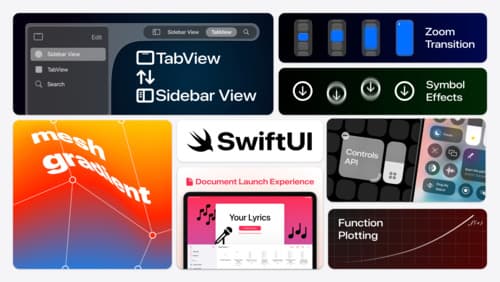
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
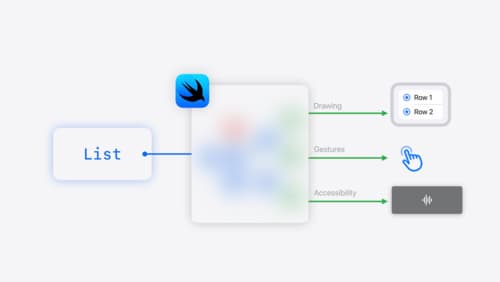
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
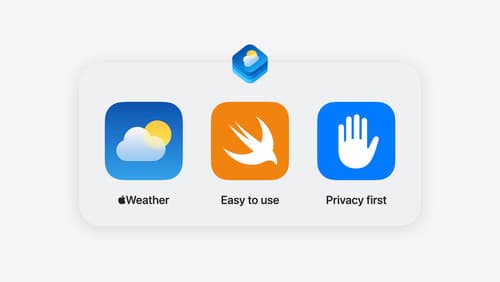
Bring context to today’s weather
Harness the power of WeatherKit to get detailed weather forecast data such as precipitation amounts by type, cloud cover by altitude, or maximum wind speed. Find out how you can summarize weather by different parts of the day and highlight significant upcoming changes to temperature or precipitation. Understand how you can compare current weather to the past through our Historical Comparisons dataset and dive into historical weather statistics for any location in the world. We’ll also explore how you can do all of this faster with our Swift and REST APIs.
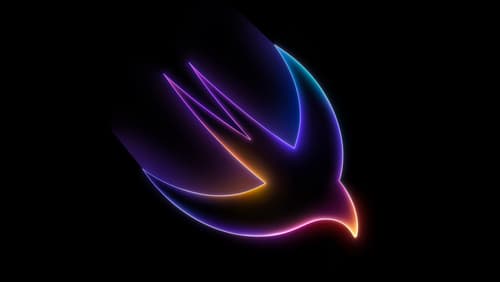
Platforms State of the Union
Discover the newest advancements on Apple platforms.
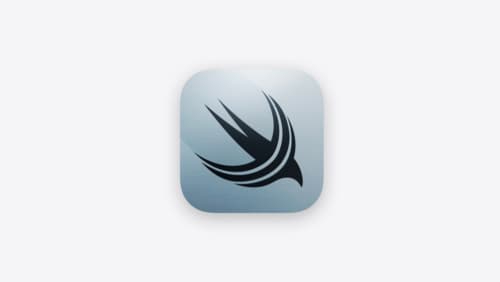
What’s new in SwiftData
SwiftData makes it easy to add persistence to your app with its expressive, declarative API. Learn about refinements to SwiftData, including compound uniqueness constraints, faster queries with #Index, queries in Xcode previews, and rich predicate expressions. Join us to explore how you can use all of these features to express richer models and improve performance in your app. To discover how to build a custom data store or use the history API in SwiftData, watch “Create a custom data store with SwiftData” and “Track model changes with SwiftData history”.