How can I write something to the database in a view model using SwiftData
Generated on 8/18/2024
1 search
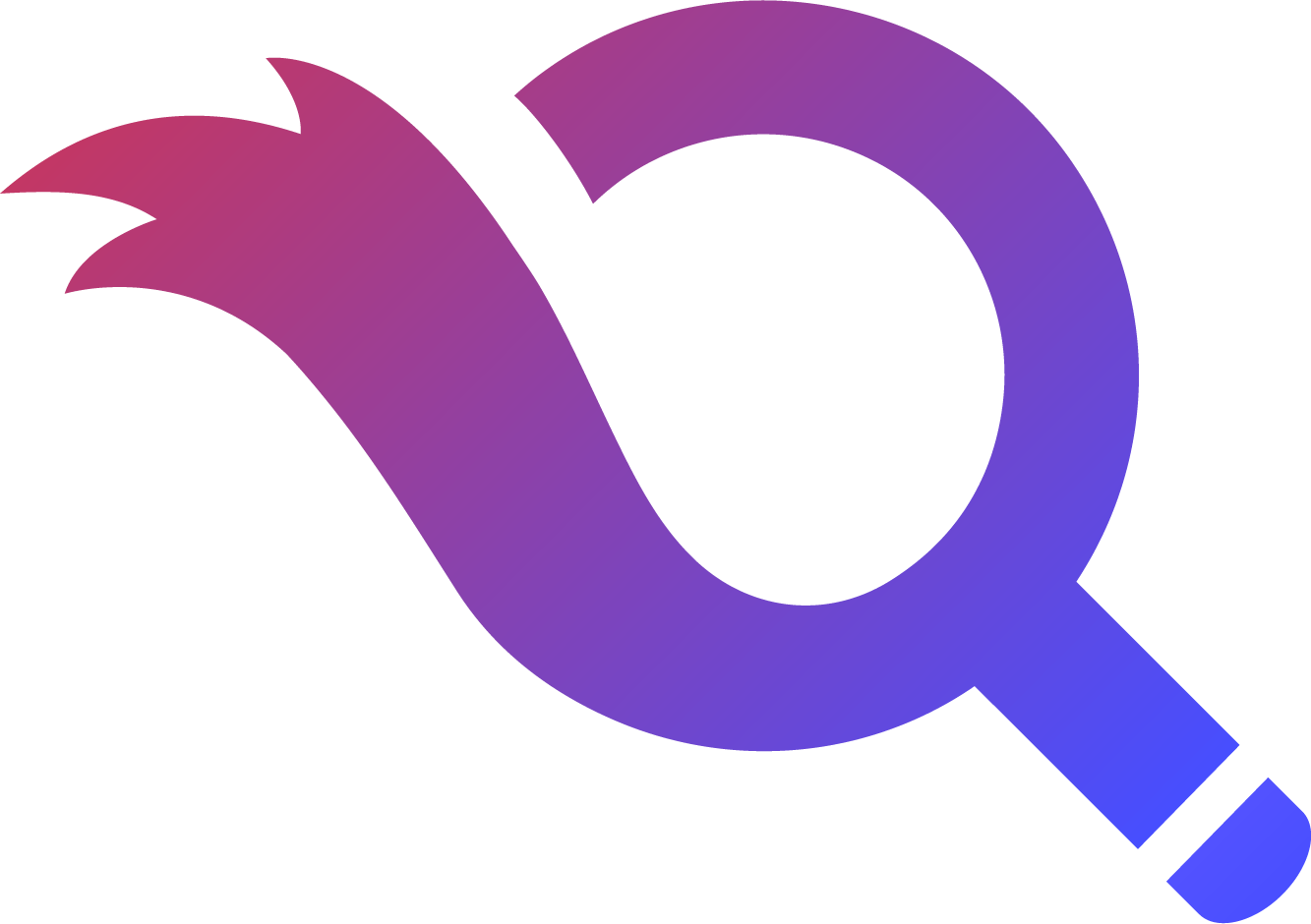
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To write something to the database in a view model using SwiftData, you can follow these steps:
-
Import SwiftData Framework: First, ensure that you have imported the SwiftData framework in your project.
-
Define Your Model: Use the model macro to define your data model. This macro helps in setting up the schema for your data.
-
Set Up Model Container: Use the model container modifier on your Window group to inform the view hierarchy about your model. This allows your views to interact with the data model.
-
Use Model Context: The model context is used to read and write data. You can use it to perform operations like saving new data to the database.
Here's a basic example of how you might implement this in a SwiftUI view model:
import SwiftData
@Model
class Trip {
var destination: String
var date: Date
init(destination: String, date: Date) {
self.destination = destination
self.date = date
}
}
struct ContentView: View {
@Environment(\.modelContext) private var modelContext
var body: some View {
Button("Add Trip") {
let newTrip = Trip(destination: "Paris", date: Date())
modelContext.insert(newTrip)
try? modelContext.save()
}
}
}
In this example, a new Trip
object is created and inserted into the model context. The save()
method is then called to persist the changes to the database.
For more detailed information, you can refer to the session What’s new in SwiftData (00:01:01) which covers the adoption of SwiftData and how to set up your models and containers.
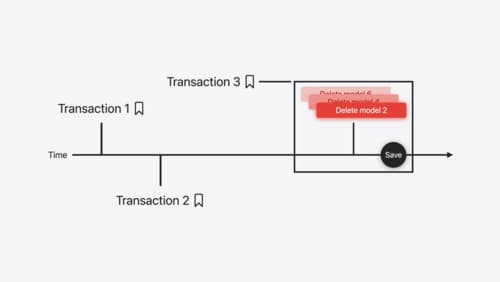
Track model changes with SwiftData history
Reveal the history of your model’s changes with SwiftData! Use the history API to understand when data store changes occurred, and learn how to use this information to build features like remote server sync and out-of-process change handing in your app. We’ll also cover how you can build support for the history API into a custom data store.
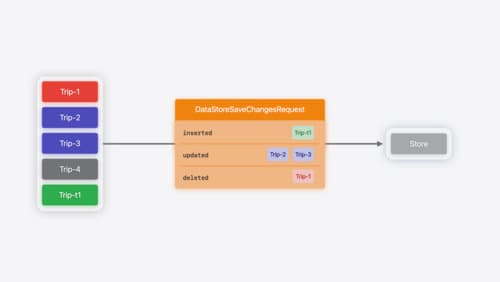
Create a custom data store with SwiftData
Combine the power of SwiftData’s expressive, declarative modeling API with your own persistence backend. Learn how to build a custom data store and explore how to progressively add persistence features in your app. To get the most out of this session, watch “Meet SwiftData” and “Model your schema with SwiftData” from WWDC23.
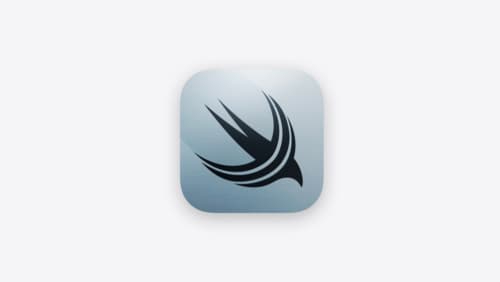
What’s new in SwiftData
SwiftData makes it easy to add persistence to your app with its expressive, declarative API. Learn about refinements to SwiftData, including compound uniqueness constraints, faster queries with #Index, queries in Xcode previews, and rich predicate expressions. Join us to explore how you can use all of these features to express richer models and improve performance in your app. To discover how to build a custom data store or use the history API in SwiftData, watch “Create a custom data store with SwiftData” and “Track model changes with SwiftData history”.
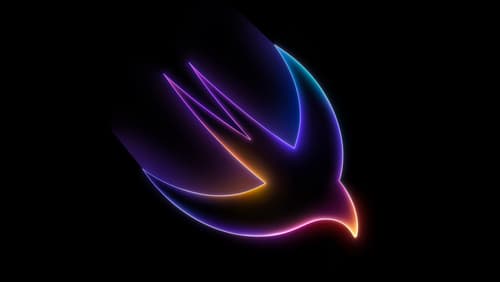
Platforms State of the Union
Discover the newest advancements on Apple platforms.