how to create a log in with apple in swiftui
Generated on 8/18/2024
1 search
To create a "Log in with Apple" feature in SwiftUI, you would typically use the ASAuthorizationAppleIDButton
from the AuthenticationServices
framework. This button provides a standard interface for users to log in with their Apple ID. Here's a basic example of how you might implement this in SwiftUI:
import SwiftUI
import AuthenticationServices
struct ContentView: View {
var body: some View {
SignInWithAppleButton(
.signIn,
onRequest: { request in
// Configure the request here
request.requestedScopes = [.fullName, .email]
},
onCompletion: { result in
switch result {
case .success(let authorization):
// Handle successful authorization
if let appleIDCredential = authorization.credential as? ASAuthorizationAppleIDCredential {
let userIdentifier = appleIDCredential.user
let fullName = appleIDCredential.fullName
let email = appleIDCredential.email
// Use the credentials as needed
}
case .failure(let error):
// Handle error
print("Authorization failed: \(error.localizedDescription)")
}
}
)
.signInWithAppleButtonStyle(.black)
.frame(width: 280, height: 45)
}
}
This code snippet creates a "Sign in with Apple" button using SwiftUI. When the button is pressed, it initiates an authorization request. You can configure the request to ask for the user's full name and email. The completion handler processes the result, handling both success and failure cases.
For more detailed guidance on using SwiftUI, you might want to check out the session SwiftUI essentials from WWDC 2024, which covers the fundamentals of views and built-in capabilities across all platforms.
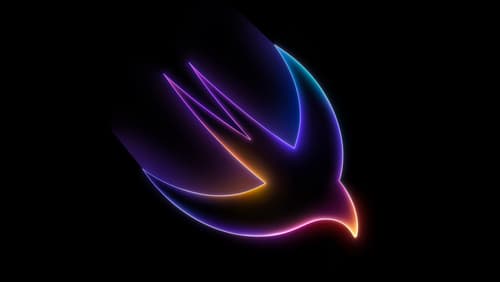
Platforms State of the Union
Discover the newest advancements on Apple platforms.
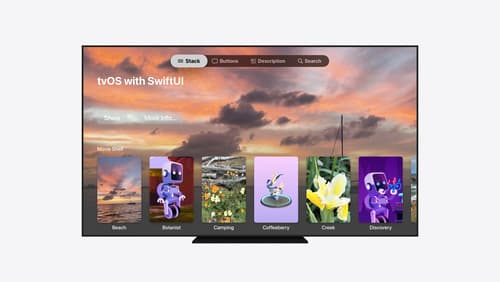
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
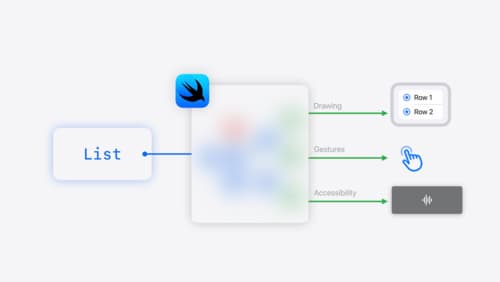
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.