how to write a unit test with swift testing, give me an example please.
Generated on 8/21/2024
1 search
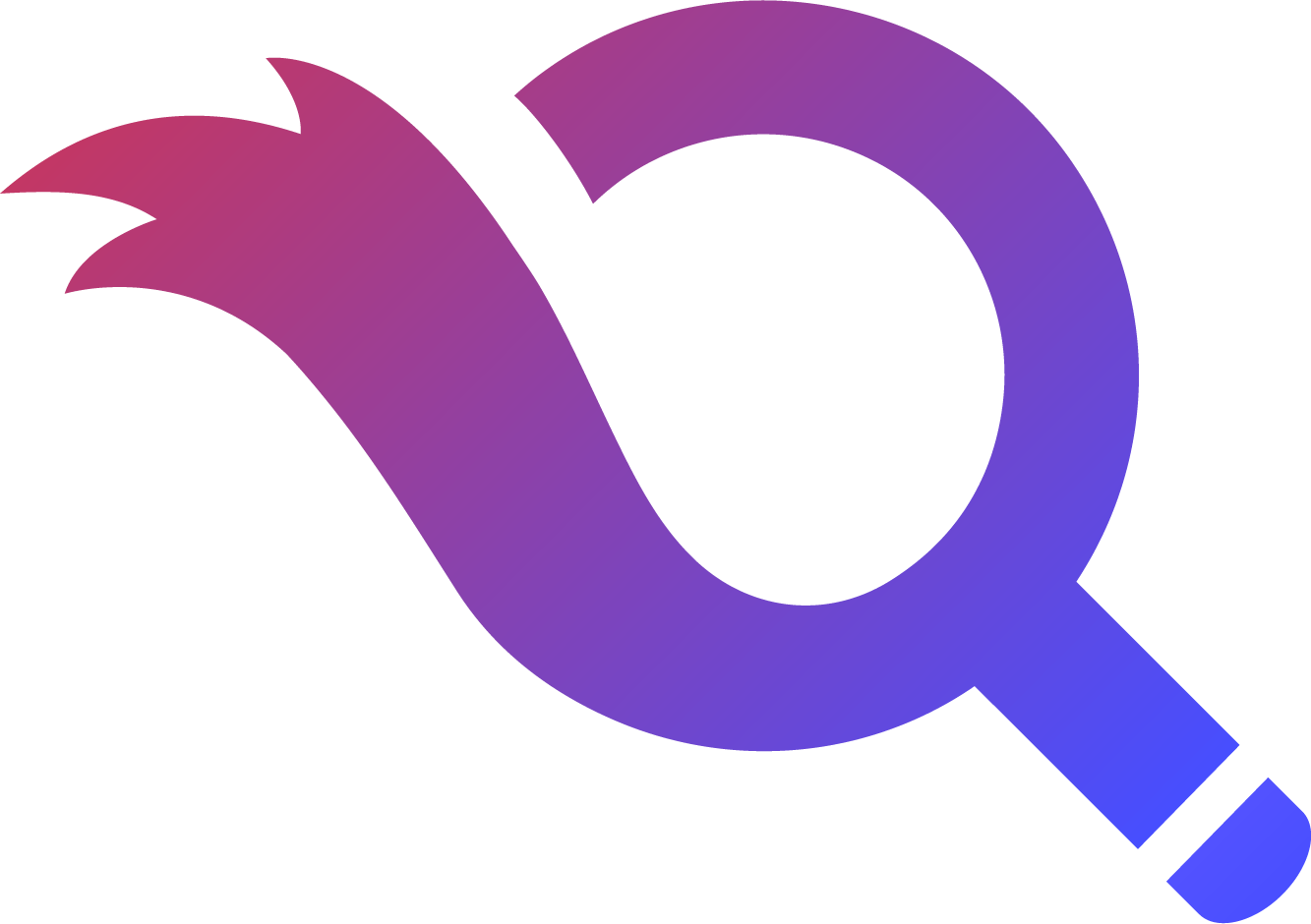
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To write a unit test using Swift Testing, you can follow these steps. Swift Testing is a new framework introduced by Apple, designed to be expressive and easy to use. Here's a simple example to get you started:
-
Create a Test Target: First, ensure you have a test target in your Xcode project. You can add one by choosing
File > New > Target
and selectingUnit Testing Bundle
. -
Import the Testing Module: In your test file, import the Swift Testing framework.
-
Write a Test Function: Define a function and use the
@Test
attribute to mark it as a test. This attribute tells Xcode that the function is a test case. -
Use the
expect
Macro: Within your test function, use theexpect
macro to evaluate expressions and verify that your code behaves as expected.
Here's an example of a simple test:
import SwiftTesting
@Test
func testAddition() {
let sum = 2 + 2
expect(sum == 4, "Sum should be 4")
}
In this example, the testAddition
function checks if the sum of 2 and 2 equals 4. The expect
macro is used to assert that the condition is true.
For more detailed information and advanced features like parameterized tests, you can refer to the session Meet Swift Testing from WWDC 2024. This session covers the building blocks of Swift Testing and provides insights into writing expressive and efficient tests.
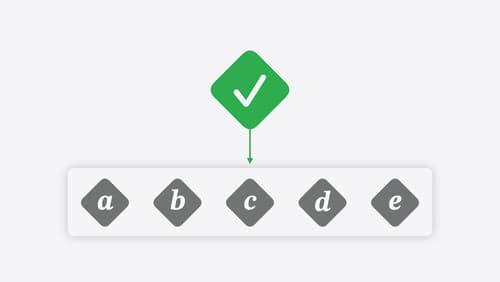
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
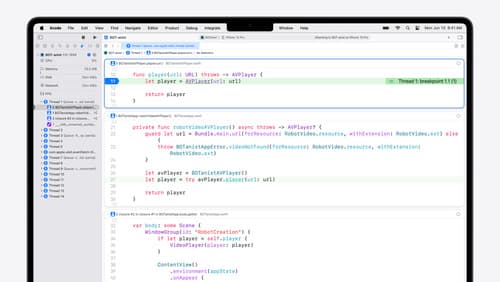
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.
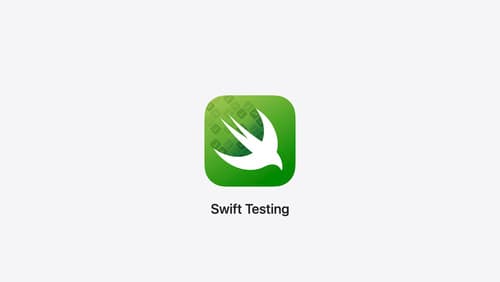
Meet Swift Testing
Introducing Swift Testing: a new package for testing your code using Swift. Explore the building blocks of its powerful new API, discover how it can be applied in common testing workflows, and learn how it relates to XCTest and open source Swift.
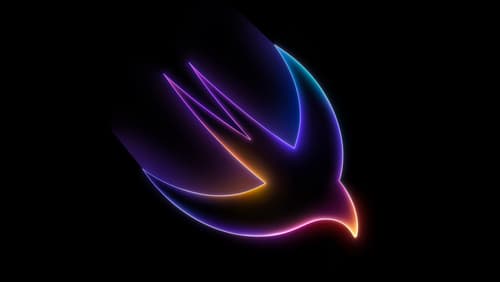
Platforms State of the Union
Discover the newest advancements on Apple platforms.
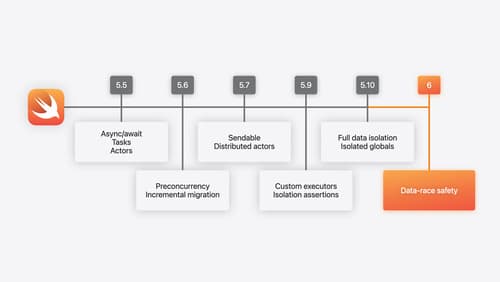
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.