how to use decodable with enum?
Generated on 8/22/2024
1 search
To use Decodable
with an enum in Swift, you can leverage Swift's powerful enum capabilities, which allow you to represent a choice between different cases, potentially with associated values. Here's a general approach to making an enum conform to Decodable
:
-
Define the Enum: Create your enum with cases. If needed, include associated values for each case.
-
Conform to Decodable: Implement the
Decodable
protocol for your enum. This typically involves writing a custom initializer that decodes the enum from aDecoder
. -
Use a Switch Statement: Within the initializer, use a switch statement to handle different cases based on the data being decoded.
Here's a simple example:
enum MyEnum: Decodable {
case on
case off
case level(Int)
case color(hue: Int, saturation: Int)
enum CodingKeys: String, CodingKey {
case type
case value
}
init(from decoder: Decoder) throws {
let container = try decoder.container(keyedBy: CodingKeys.self)
let type = try container.decode(String.self, forKey: .type)
switch type {
case "on":
self = .on
case "off":
self = .off
case "level":
let value = try container.decode(Int.self, forKey: .value)
self = .level(value)
case "color":
let hue = try container.decode(Int.self, forKey: .value)
let saturation = try container.decode(Int.self, forKey: .value)
self = .color(hue: hue, saturation: saturation)
default:
throw DecodingError.dataCorruptedError(forKey: .type, in: container, debugDescription: "Invalid type")
}
}
}
This example demonstrates how to decode an enum with different cases, some of which have associated values. The use of a switch statement allows you to handle each case appropriately, leveraging Swift's pattern matching capabilities.
For more detailed information on using enums in Swift, you might find the session Go small with Embedded Swift helpful, as it discusses the power and expressiveness of Swift enums.
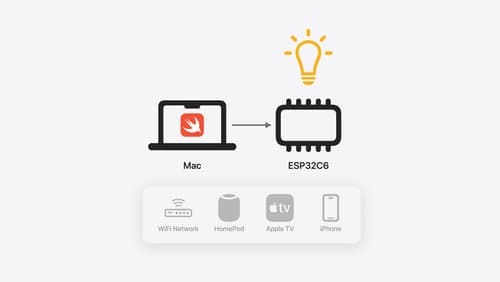
Go small with Embedded Swift
Embedded Swift brings the safety and expressivity of Swift to constrained environments. Explore how Embedded Swift runs on a variety of microcontrollers through a demonstration using an off-the-shelf Matter device. Learn how the Embedded Swift subset packs the benefits of Swift into a tiny footprint with no runtime, and discover plenty of resources to start your own Embedded Swift adventure.
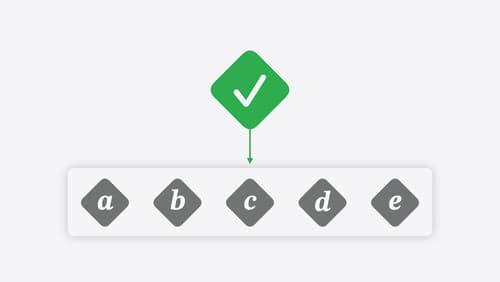
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
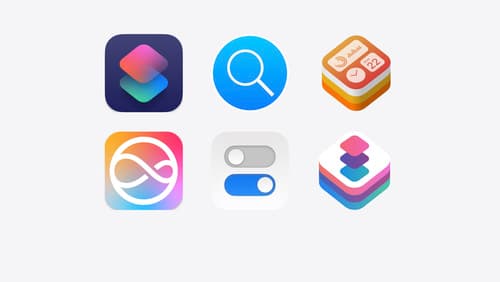
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.
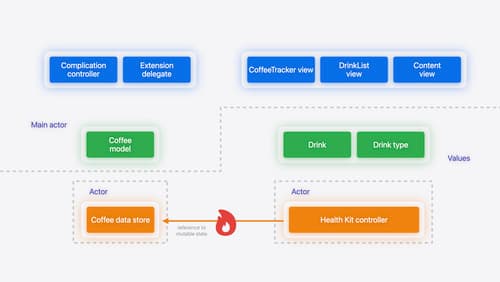
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
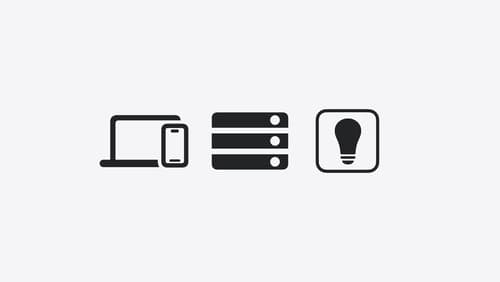
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.