mutating struct vs class
Generated on 8/22/2024
1 search
In Swift, the choice between using a struct or a class often comes down to how you want to manage data and its mutability.
Structs
- Value Types: Structs are value types, which means that when you assign a struct to a new variable or pass it to a function, you are working with a copy of the original data. This is beneficial for ensuring that data is not accidentally modified elsewhere in your code.
- Inline Storage: Structs use inline storage, which can be more efficient for small data types as it avoids heap allocation. However, copying large structs can be costly in terms of performance because it involves copying all stored properties.
- Mutability Control: You can control the mutability of structs by using
let
andvar
. A struct declared withlet
is immutable, meaning its properties cannot be changed after initialization.
Classes
- Reference Types: Classes are reference types, meaning that when you assign a class instance to a new variable or pass it to a function, you are working with a reference to the same instance. This is useful for shared mutable state.
- Out of Line Storage: Classes use out of line storage, which means they store their data on the heap. This can be more efficient for large data types as it avoids copying the data itself, only the reference.
- Automatic Reference Counting (ARC): Swift uses ARC to manage memory for class instances, ensuring that objects are deallocated when there are no more references to them. However, care must be taken to avoid reference cycles, which can prevent deallocation.
Use Cases
- Use Structs: When you want to ensure data integrity and immutability, or when working with small data types that benefit from inline storage.
- Use Classes: When you need shared mutable state, identity, or inheritance.
For more detailed insights, you can refer to the session Explore Swift performance (13:57) and A Swift Tour: Explore Swift’s features and design (11:58).
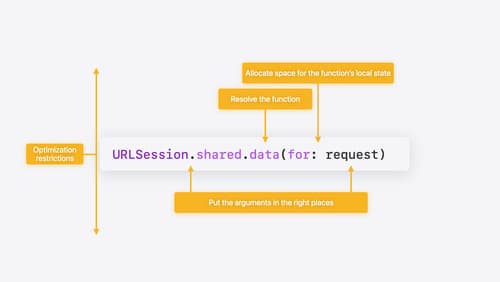
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
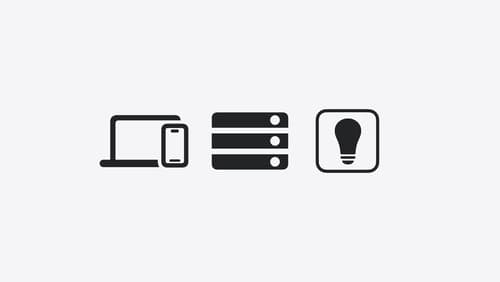
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
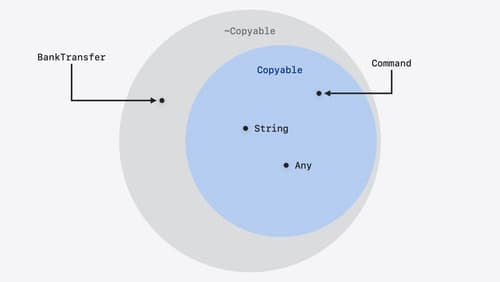
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.