How to configure AVQueuePlayer?
Generated on 9/2/2024
1 search
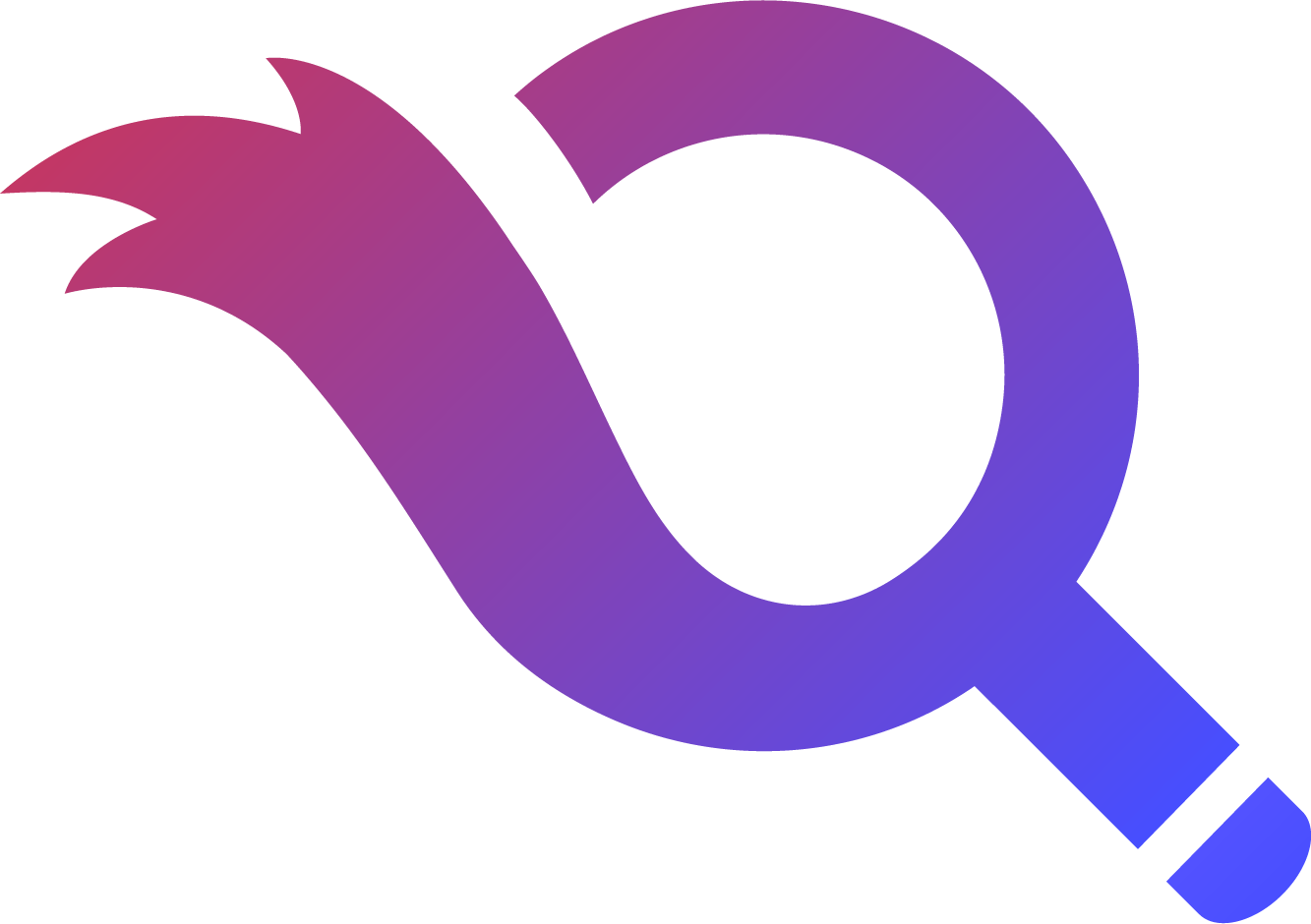
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To configure an AVQueuePlayer
, you can follow these general steps:
-
Initialize the AVQueuePlayer: Create an instance of
AVQueuePlayer
with an array ofAVPlayerItem
objects. EachAVPlayerItem
represents a piece of media you want to play in sequence.let playerItem1 = AVPlayerItem(url: URL(string: "https://example.com/video1.mp4")!) let playerItem2 = AVPlayerItem(url: URL(string: "https://example.com/video2.mp4")!) let queuePlayer = AVQueuePlayer(items: [playerItem1, playerItem2])
-
Add or Remove Items: You can add or remove items from the queue as needed using
insert(_:after:)
to add andremove(_:completionHandler:)
to remove.let playerItem3 = AVPlayerItem(url: URL(string: "https://example.com/video3.mp4")!) queuePlayer.insert(playerItem3, after: playerItem1)
-
Control Playback: Use methods like
play()
,pause()
, andadvanceToNextItem()
to control playback.queuePlayer.play()
-
Observe Player Status: You can observe the player's status and the current item to handle events like playback completion or errors.
queuePlayer.addObserver(self, forKeyPath: "status", options: [.new, .initial], context: nil)
-
Handle Events: Implement the observer method to respond to changes in the player's status or the current item.
override func observeValue(forKeyPath keyPath: String?, of object: Any?, change: [NSKeyValueChangeKey : Any]?, context: UnsafeMutableRawPointer?) { if keyPath == "status" { if queuePlayer.status == .readyToPlay { // Player is ready to play } else if queuePlayer.status == .failed { // Handle error } } }
For more detailed information on AVFoundation and media playback, you might find the session Discover media performance metrics in AVFoundation helpful, especially the section on subscribing to events.
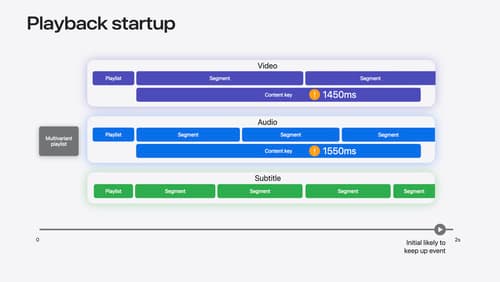
Discover media performance metrics in AVFoundation
Discover how you can monitor, analyze, and improve user experience with the new media performance APIs. Explore how to monitor AVPlayer performance for HLS assets using different AVMetricEvents, and learn how to use these metrics to understand and triage player performance issues.
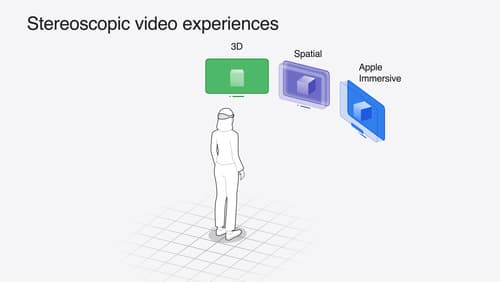
Build compelling spatial photo and video experiences
Learn how to adopt spatial photos and videos in your apps. Explore the different types of stereoscopic media and find out how to capture spatial videos in your iOS app on iPhone 15 Pro. Discover the various ways to detect and present spatial media, including the new QuickLook Preview Application API in visionOS. And take a deep dive into the metadata and stereo concepts that make a photo or video spatial.
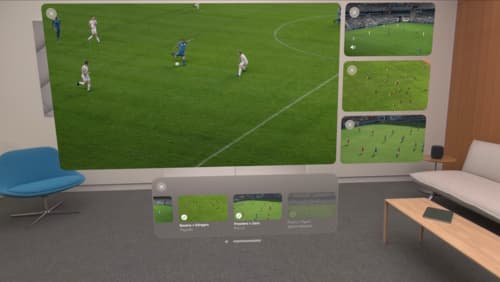
Explore multiview video playback in visionOS
Learn how AVExperienceController can enable playback of multiple videos on Apple Vision Pro. Review best practices for adoption and explore great use cases, like viewing a sports broadcast from different angles or watching multiple games simultaneously. And discover how to design a compelling and intuitive multiview experience in your app.
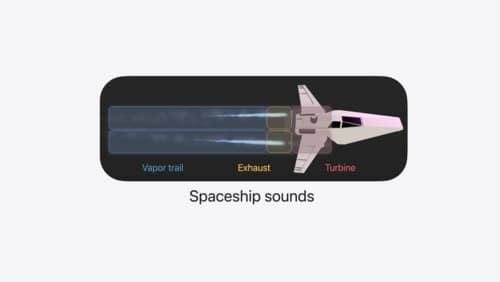
Enhance your spatial computing app with RealityKit audio
Elevate your spatial computing experience using RealityKit audio. Discover how spatial audio can make your 3D immersive experiences come to life. From ambient audio, reverb, to real-time procedural audio that can add character to your 3D content, learn how RealityKit audio APIs can help make your app more engaging.