what is the difference between weak and unowned?
Generated on 9/6/2024
1 search
In Swift, weak and unowned references are used to avoid strong reference cycles, which can prevent objects from being deallocated. Here's a summary of their differences and when to use them:
-
Weak References:
- Weak references are always optional types. They become
nil
after their destination objects are deinitialized. - They are useful when you don't know how long the destination will live, as they allow the destination to be deallocated when no strong references exist.
- Weak references come with some overhead because Swift allocates a weak reference storage for the destination object the first time it's weakly referenced.
- They are a good default choice when you need to avoid strong reference cycles.
- Weak references are always optional types. They become
-
Unowned References:
- Unowned references directly hold their destination objects, meaning they don't use extra memory and are faster to access than weak references.
- They can be non-optional and constant, but they must always point to a valid object. If the object is deinitialized, accessing an unowned reference will cause a crash.
- Use unowned references when you can guarantee that the reference will not outlive its destination, saving memory and time.
For more detailed information, you can refer to the session Analyze heap memory (26:51) from WWDC 2024, which discusses the performance comparison between weak and unowned references.
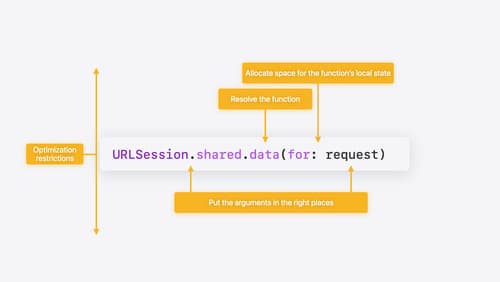
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
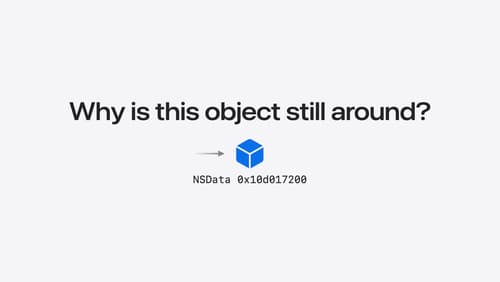
Analyze heap memory
Dive into the basis for your app’s dynamic memory: the heap! Explore how to use Instruments and Xcode to measure, analyze, and fix common heap issues. We’ll also cover some techniques and best practices for diagnosing transient growth, persistent growth, and leaks in your app.
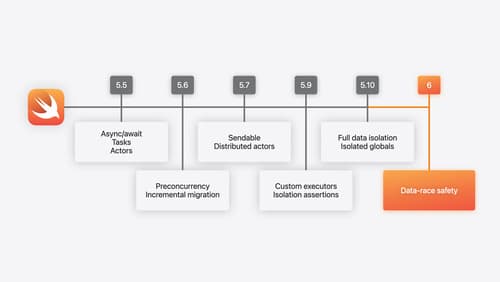
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
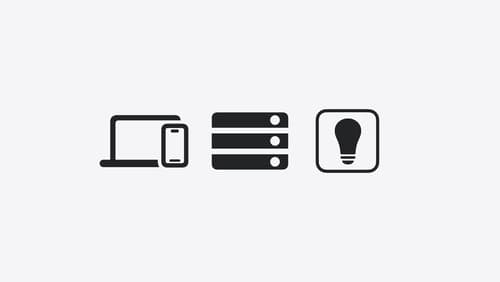
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.