list out what's new in SwiftUI since ios 15, remember to include code sample and what ios version
Asked on 09/06/2024
1 search
Since iOS 15, SwiftUI has introduced several new features and improvements. Here are some of the highlights:
-
Custom Containers: SwiftUI now allows for the creation of custom containers, providing more flexibility in how you structure your UI components.
-
Mesh Gradients: A new feature that lets you create beautiful, complex gradients for your UI.
-
Scrolling Customizations: You can now have fine-grained control over scroll views, including reacting to changes in content offsets and sizes. This allows for features like auto-playing videos when they come into view.
-
Custom Animations: SwiftUI now supports setting up animations on UIKit or AppKit views, which can be driven by SwiftUI, including fully custom animations.
-
Improved Interoperability: Gesture recognition has been factored out of UIKit, allowing you to use any built-in or custom UI gesture recognizer in your SwiftUI view hierarchy.
-
Accessibility Enhancements: SwiftUI has added new accessibility features, such as conditional modifier support and app intent-based accessibility actions.
-
Xcode Previews: A new dynamic linking architecture in Xcode Previews allows for a smoother workflow by using the same build artifacts for previews and when you build and run.
-
Text Renderer API: This new API enables advanced visual effects and animations for text.
Here is a simple code example demonstrating the use of mesh gradients in SwiftUI:
import SwiftUI
struct ContentView: View {
var body: some View {
Rectangle()
.fill(
LinearGradient(
gradient: Gradient(colors: [.blue, .purple, .pink]),
startPoint: .topLeading,
endPoint: .bottomTrailing
)
)
.frame(width: 200, height: 200)
}
}
For more detailed information, you can refer to the What’s new in SwiftUI session.
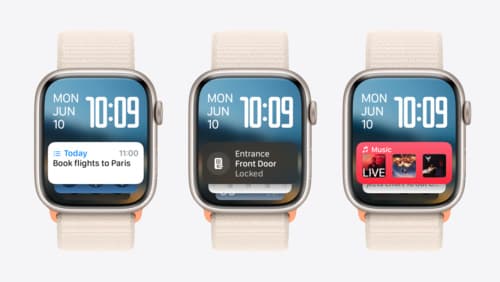
What’s new in watchOS 11
Explore new opportunities on Apple Watch, including bringing Double Tap support to your watchOS app, making your Smart Stack widgets even more relevant and interactive, and displaying your iOS Live Activities in the Smart Stack.
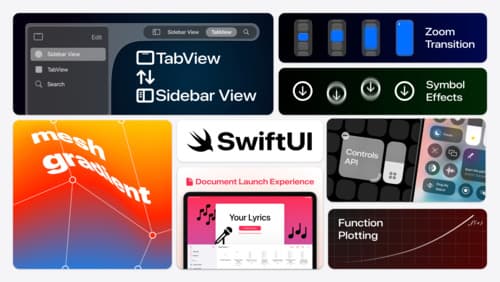
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
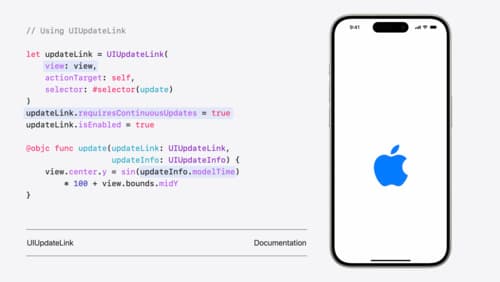
What’s new in UIKit
Explore everything new in UIKit, including tab and document launch experiences, transitions, and text and input changes. We’ll also discuss better-than-ever interoperability between UIKit and SwiftUI animations and gestures, as well as general improvements throughout UIKit.