How to display list in SwiftUI
Generated on 9/6/2024
1 search
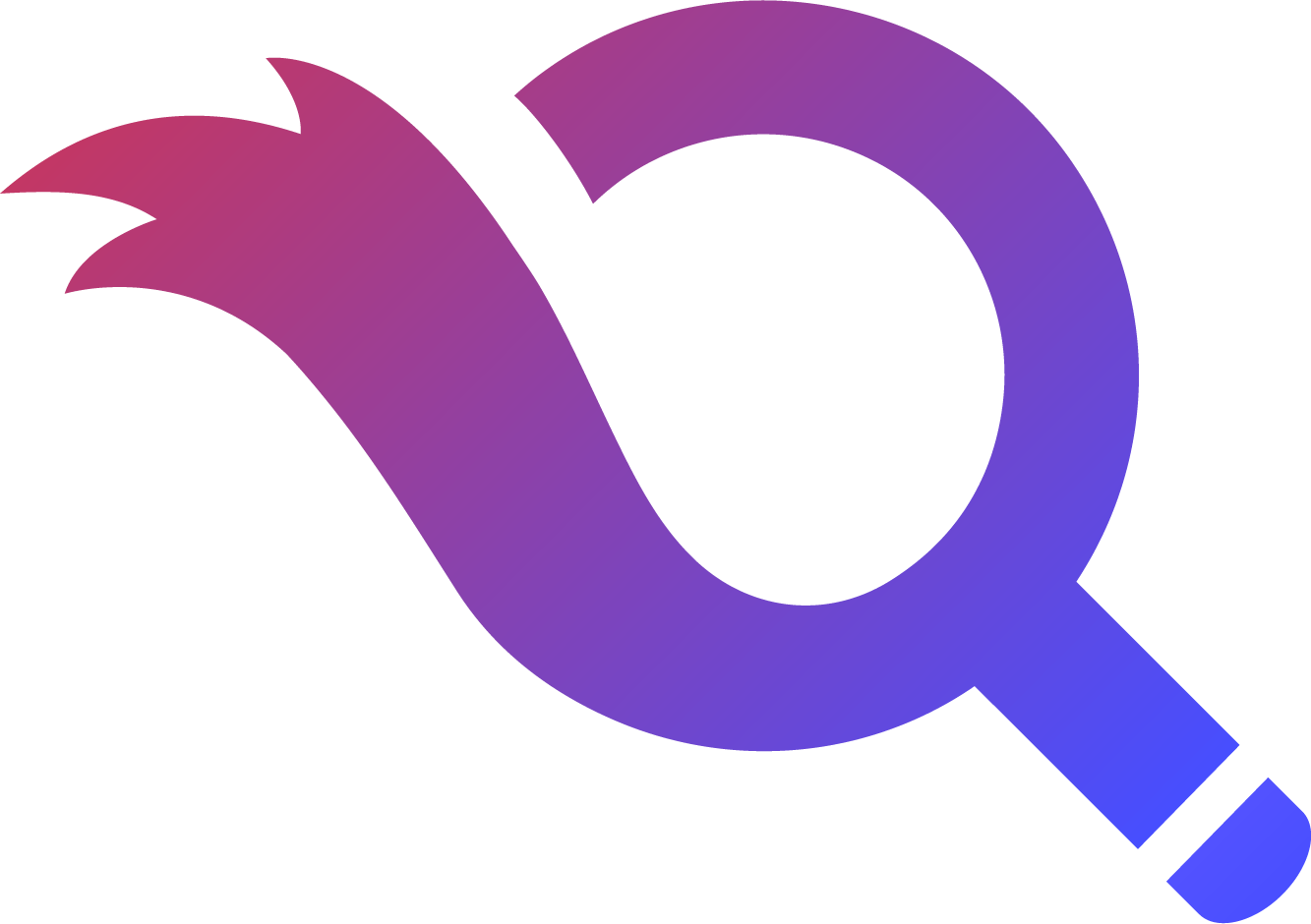
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To display a list in SwiftUI, you can use the List
view, which is a powerful tool for view composition. The List
initializer can take a collection parameter, which is a convenience for creating a ForEach
view. The ForEach
view generates views for each element in a collection and provides those to its container. This allows you to create advanced constructions, such as multiple collections of data organized into sections.
Here's a basic example of how you might use a List
in SwiftUI:
struct ContentView: View {
let pets = ["Whiskers", "Rufus", "Bubbles"]
var body: some View {
List(pets, id: \.self) { pet in
Text(pet)
}
}
}
In this example, the List
is initialized with a collection of pet names. The ForEach
view is used implicitly to generate a Text
view for each pet name in the list.
Additionally, you can customize lists using view modifiers, such as adding swipe actions to each row. SwiftUI's declarative system ensures that when your view state changes over time, the UI is automatically kept up to date.
For more detailed information, you can refer to the session SwiftUI essentials (07:55) which covers the fundamentals of using lists in SwiftUI.
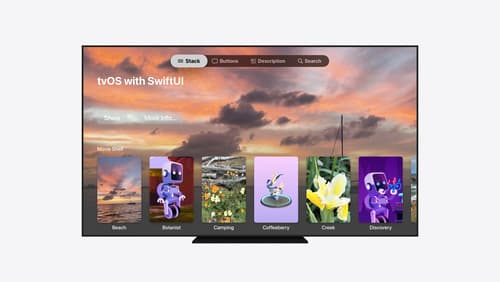
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
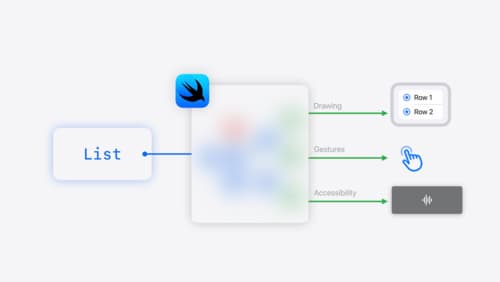
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
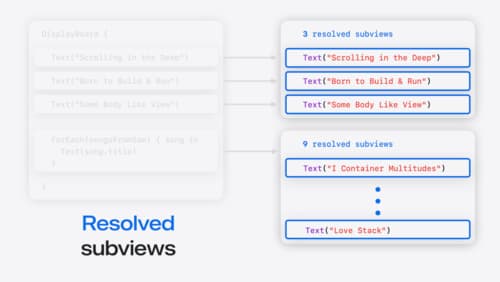
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.