I have created objective-c framework and outside xcframwork copied the resources bundles. When adding binary framework in swiftui, how should that bundle be linked or added?
Asked on 09/08/2024
1 search
To link or add a resource bundle from an Objective-C framework to a SwiftUI app, you can take advantage of SwiftUI's interoperability with other frameworks. SwiftUI allows you to incorporate UIKit or AppKit views, which can be useful for accessing resources from an Objective-C framework.
Here are some general steps you might follow:
-
Include the Framework: Ensure that your Objective-C framework is properly included in your SwiftUI project. This typically involves adding the framework to your project settings under "Linked Frameworks and Libraries."
-
Access the Bundle: You can access the resource bundle from your Objective-C framework using
Bundle
in Swift. For example, if your framework is namedMyFramework
, you can access its bundle like this:if let bundleURL = Bundle.main.url(forResource: "MyFramework", withExtension: "bundle"), let bundle = Bundle(url: bundleURL) { // Use the bundle to access resources }
-
Use the Resources: Once you have access to the bundle, you can load resources such as images, strings, or other assets using the appropriate APIs.
For more detailed guidance, you might want to refer to the session on SwiftUI essentials which discusses SDK interoperability and how SwiftUI can work with other frameworks.
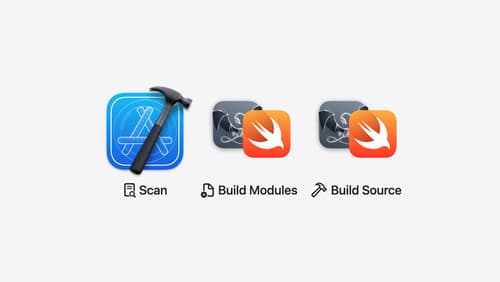
Demystify explicitly built modules
Explore how builds are changing in Xcode 16 with explicitly built modules. Discover how modules are used to build your code, how explicitly built modules improve transparency in compilation tasks, and how you can optimize your build by sharing modules across targets.
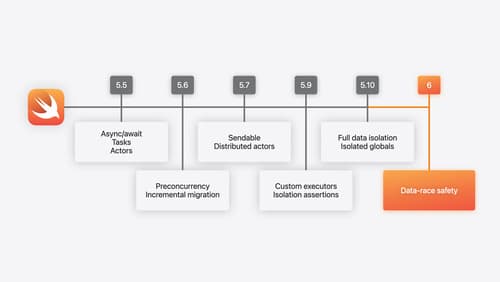
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
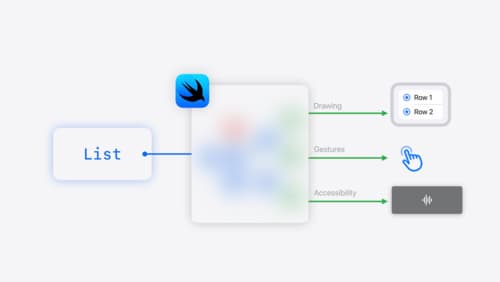
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.