how to properly use async func inside a completion-based method?
Asked on 09/13/2024
1 search
To properly use an async
function inside a completion-based method in Swift, you can take advantage of Swift's concurrency features. If the code you're working with uses a completion handler and an async
overload is not available, you can convert it to an expression that can be awaited using withCheckedContinuation
or withCheckedThrowingContinuation
. This allows you to bridge the gap between completion handlers and async/await
syntax.
Here's a brief overview of how you can achieve this:
-
Check for Async Overloads: First, see if Swift provides an
async
overload for the completion handler-based method. If it does, you can directly use theasync
version. -
Use Continuations: If no
async
overload is available, usewithCheckedContinuation
orwithCheckedThrowingContinuation
to wrap the completion handler. This will allow you to await the result of the asynchronous operation. -
Handle Multiple Callbacks: If the callback may fire more than once, such as in an event handler, ensure that your code safely handles concurrency. Swift 6 introduces stricter concurrency checks to prevent data races, so be mindful of how you manage shared state.
For more detailed information, you can refer to the session Go further with Swift Testing (24:51) which discusses handling asynchronous conditions in testing, and the session A Swift Tour: Explore Swift’s features and design (18:33) which covers concurrency basics in Swift.
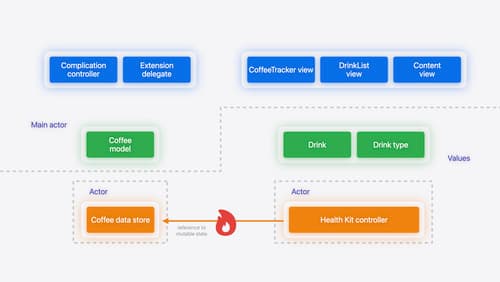
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
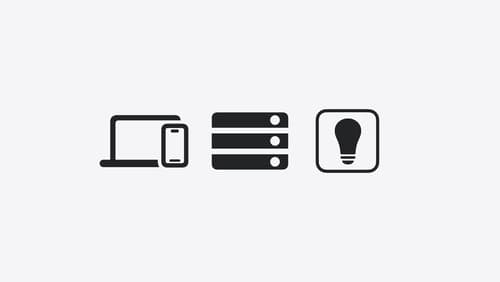
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
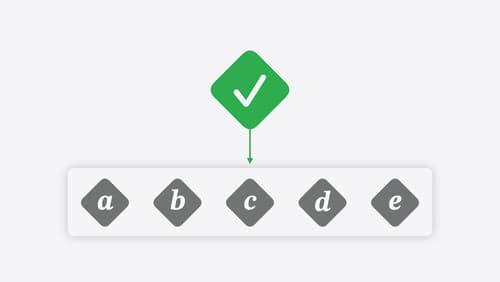
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.