geef een voorbeeld van async wait
Generated on 9/24/2024
1 search
In Swift, the async
and await
keywords are used to handle asynchronous operations. An async
function is one that can pause its execution to wait for a result, allowing other tasks to run in the meantime. The await
keyword is used when calling an async
function to indicate that the function may suspend at that point.
Here's a simple example of how async
and await
can be used in Swift:
func fetchData() async -> String {
// Simulate a network call
await Task.sleep(2 * 1_000_000_000) // Sleep for 2 seconds
return "Data fetched"
}
func processData() async {
let data = await fetchData()
print(data)
}
In this example, fetchData
is an async
function that simulates a network call by sleeping for 2 seconds. The processData
function calls fetchData
using the await
keyword, indicating that it will wait for fetchData
to complete before continuing.
For more detailed information on async functions and their performance, you can refer to the session Explore Swift performance (24:33) from WWDC 2024.
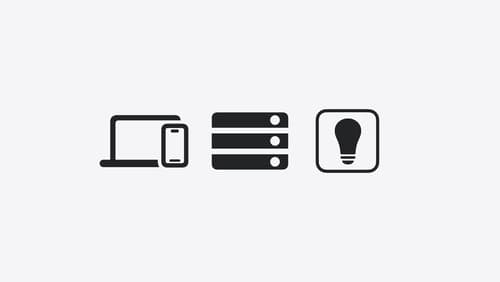
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
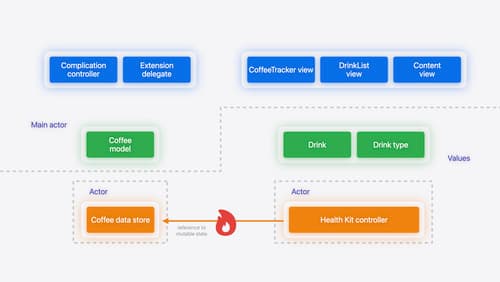
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
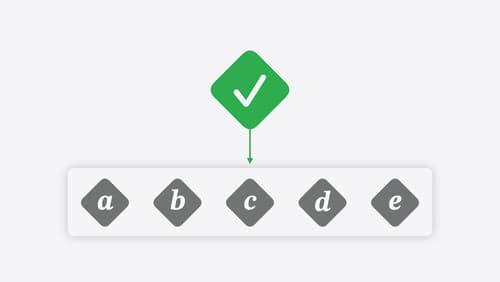
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
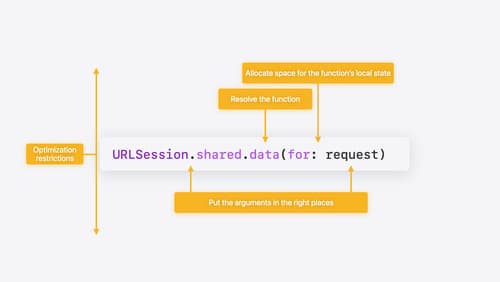
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.