can you teach me iOS Concurrency in great detail? like I'm a student that want to know everything, every possible API that Apple provide and how they work, their trade-offs, how to decide which one to use etc
Generated on 9/26/2024
1 search
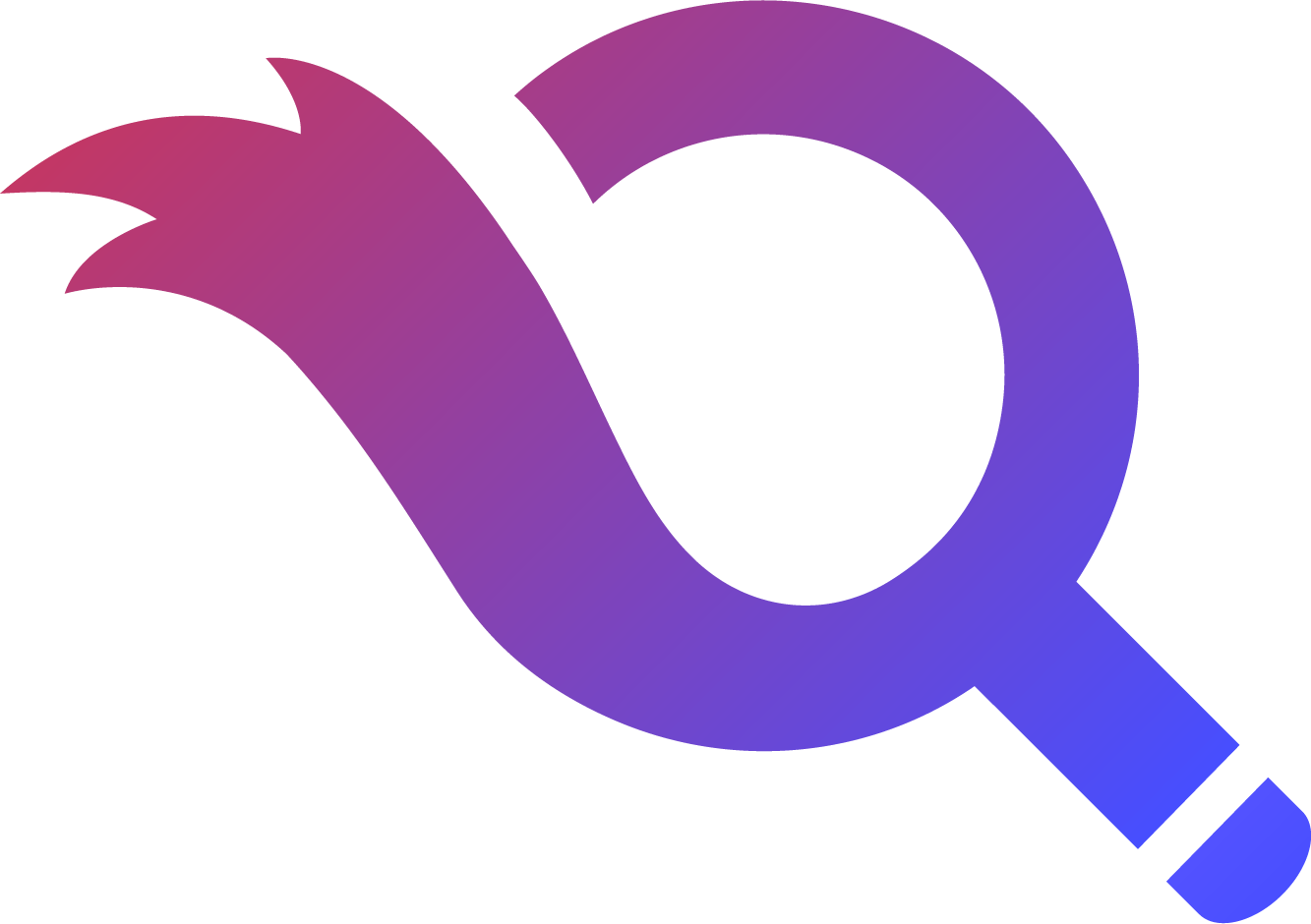
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To learn about iOS concurrency in detail, you can explore the sessions from Apple's WWDC that cover Swift concurrency features and their applications. Here are some key points and resources from the sessions:
-
Concurrency in Swift:
- The fundamental unit of concurrency in Swift is a task, which represents an independent concurrent execution context. Tasks are lightweight and can be created in large numbers. They can perform asynchronous operations and suspend to yield the CPU to other tasks when waiting for an operation to complete.
- Swift's concurrency model includes features like
async/await
and actors, which help manage concurrent code execution and data race safety.
-
Migrating to Swift 6:
- Swift 6 introduces enhanced data race safety, allowing developers to leverage concurrency without fear of introducing new data races.
- The migration to Swift 6 involves adopting concurrency features and enabling strict concurrency checking to ensure data isolation and safety.
- The use of actors in Swift 6 helps in organizing concurrency by isolating state and ensuring that only one task can access the state at a time.
-
Concurrency Architecture:
- In a typical app, UI views and models can be set to run on the main actor, while background operations are performed on dedicated actors. This helps in organizing the concurrency architecture clearly.
- Communication between actors is done using thread-safe value types and Swift's
async/await
feature.
-
Handling Delegate Callbacks:
- When dealing with delegate callbacks, it's important to understand the concurrency guarantees provided by the callback. Some callbacks guarantee execution on the main thread, which is crucial for UI updates.
-
Practical Considerations:
- When enabling strict concurrency checking, it's often simpler to start with the UI layer, as it typically runs on the main thread and uses APIs that guarantee main-thread execution.
- It's important to be aware of modules or libraries that haven't been updated for Swift concurrency, as they might not provide the same concurrency guarantees.
For a more comprehensive understanding, you can refer to the following sessions from WWDC 2024:
- Migrate your app to Swift 6 (Swift 6 and data-race safety)
- A Swift Tour: Explore Swift’s features and design (Concurrency)
These sessions provide insights into the concurrency model in Swift, how to migrate to Swift 6, and practical strategies for adopting concurrency features in your applications.
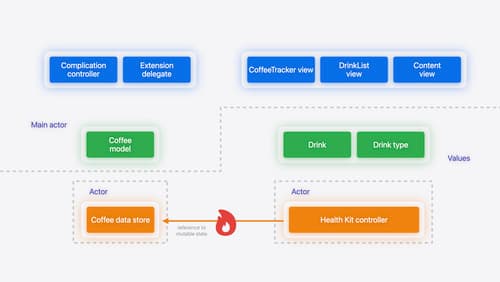
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
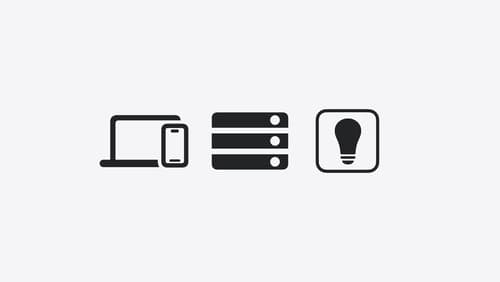
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.