give me basic setup of URLSessionWebSocketTask, to maintain a socket connection, allow for disonnection, and suport reconnecting as best practice by this framework
Asked on 10/04/2024
1 search
To set up a URLSessionWebSocketTask
for maintaining a WebSocket connection, allowing for disconnection, and supporting reconnection as a best practice, you can follow these general steps:
-
Create a URLSession and WebSocket Task:
- Initialize a
URLSession
and create aURLSessionWebSocketTask
with the desired URL.
- Initialize a
-
Connect and Listen for Messages:
- Start the WebSocket task and set up a loop to continuously receive messages. Use the
receive
method to handle incoming messages asynchronously.
- Start the WebSocket task and set up a loop to continuously receive messages. Use the
-
Send Messages:
- Use the
send
method to transmit messages over the WebSocket connection.
- Use the
-
Handle Disconnection:
- Implement a mechanism to handle disconnections gracefully. This can include listening for errors or close events and cleaning up resources.
-
Reconnect Logic:
- Implement a reconnection strategy. This could involve attempting to reconnect after a delay when a disconnection is detected. Consider using exponential backoff to manage reconnection attempts.
-
Concurrency and Thread Safety:
- Ensure that your WebSocket handling code is thread-safe. Use Swift's concurrency features, such as
async/await
, to manage asynchronous operations effectively.
- Ensure that your WebSocket handling code is thread-safe. Use Swift's concurrency features, such as
For more detailed guidance on concurrency in Swift, you might find the session A Swift Tour: Explore Swift’s features and design helpful, as it covers concurrency concepts like tasks and async/await
.
If you are interested in how concurrency is handled in Swift 6, the session Migrate your app to Swift 6 discusses delegate callbacks and concurrency, which might provide additional insights into managing asynchronous operations in your app.
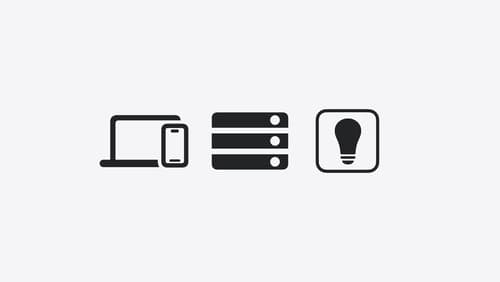
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
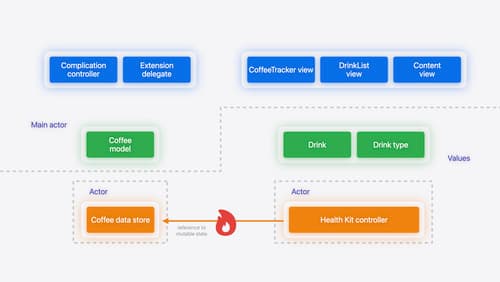
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
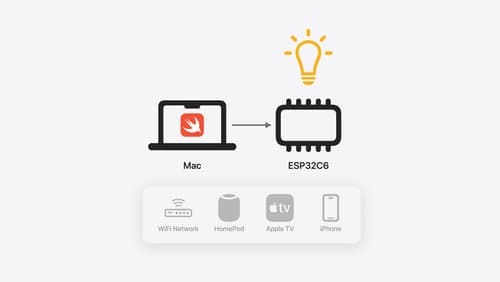
Go small with Embedded Swift
Embedded Swift brings the safety and expressivity of Swift to constrained environments. Explore how Embedded Swift runs on a variety of microcontrollers through a demonstration using an off-the-shelf Matter device. Learn how the Embedded Swift subset packs the benefits of Swift into a tiny footprint with no runtime, and discover plenty of resources to start your own Embedded Swift adventure.