how do I make a rounded rectangle in swiftui
Asked on 2024-10-24
1 search
To create a rounded rectangle in SwiftUI, you can use the RoundedRectangle
shape. Here's a simple example of how you can create a rounded rectangle with a specific corner radius:
import SwiftUI
struct ContentView: View {
var body: some View {
RoundedRectangle(cornerRadius: 25)
.fill(Color.blue)
.frame(width: 200, height: 100)
}
}
In this example, RoundedRectangle
is used to create a rectangle with rounded corners. The cornerRadius
parameter specifies the radius of the corners. The fill
modifier is used to set the color of the rectangle, and the frame
modifier sets its size.
For more advanced customization, you can explore SwiftUI's Path
and Shape
protocols, which allow for more complex shapes and designs. If you're interested in learning more about drawing paths and shapes in SwiftUI, you might want to check out the session on building a spatial drawing app with RealityKit, which discusses using SwiftUI paths for flexible shape definitions.
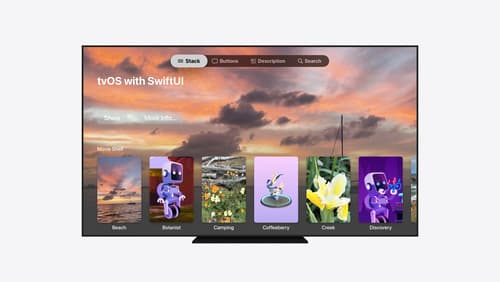
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
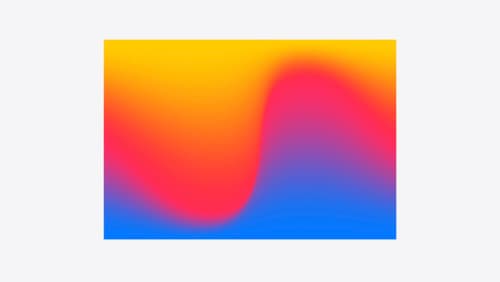
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
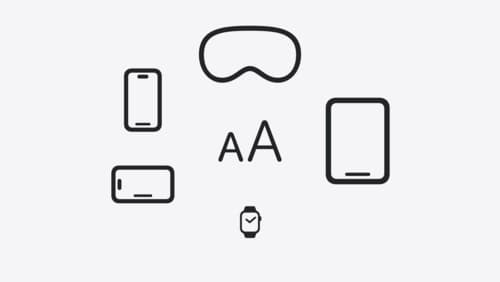
Get started with Dynamic Type
Dynamic Type lets people choose their preferred text size across the system and all of their apps. To help you get started supporting Dynamic Type, we’ll cover the fundamentals: How it works, how to find issues with scaling text in your app, and how to take practical steps using SwiftUI and UIKit to create a great Dynamic Type experience. We’ll also show how you can best use the Large Content Viewer to make navigation controls accessible to everyone.