what are best practices for converting String to a Date
Generated on 10/26/2024
1 search
To convert a String
to a Date
in Swift, you should use the DateFormatter
class, which allows you to specify the date format of the string you are trying to parse. Here are some best practices for this process:
-
Define the Date Format: Use the
dateFormat
property ofDateFormatter
to specify the format of the date string. Make sure it matches the format of the string you are trying to convert. -
Locale and Time Zone: Set the
locale
andtimeZone
properties of theDateFormatter
to ensure that the date is interpreted correctly. This is especially important if the date string includes locale-specific elements or if you need to account for time zone differences. -
Use ISO8601DateFormatter for ISO 8601 Dates: If you are working with ISO 8601 date strings, consider using
ISO8601DateFormatter
, which is specifically designed for parsing and formatting ISO 8601 dates. -
Error Handling: Always handle the possibility of a
nil
result when usingdate(from:)
, as the conversion might fail if the string does not match the expected format. -
Performance Considerations: Reuse
DateFormatter
instances when possible, as creating new instances can be expensive in terms of performance.
Here's a simple example of converting a string to a date:
let dateString = "2024-06-03"
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "yyyy-MM-dd"
dateFormatter.locale = Locale(identifier: "en_US_POSIX")
dateFormatter.timeZone = TimeZone(secondsFromGMT: 0)
if let date = dateFormatter.date(from: dateString) {
print("Converted Date: \(date)")
} else {
print("Failed to convert date")
}
This example sets up a DateFormatter
with a specific date format, locale, and time zone, and then attempts to convert a string to a Date
object.
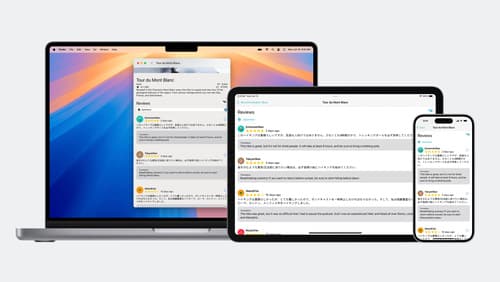
Meet the Translation API
Discover how you can translate text across different languages in your app using the new Translation framework. We’ll show you how to quickly display translations in the system UI, and how to translate larger batches of text for your app’s UI.
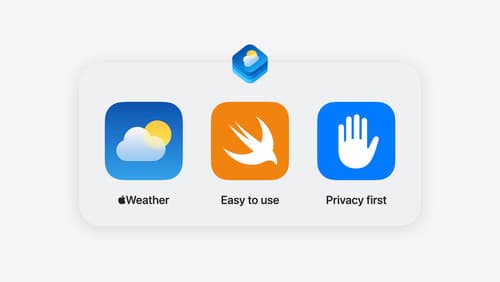
Bring context to today’s weather
Harness the power of WeatherKit to get detailed weather forecast data such as precipitation amounts by type, cloud cover by altitude, or maximum wind speed. Find out how you can summarize weather by different parts of the day and highlight significant upcoming changes to temperature or precipitation. Understand how you can compare current weather to the past through our Historical Comparisons dataset and dive into historical weather statistics for any location in the world. We’ll also explore how you can do all of this faster with our Swift and REST APIs.
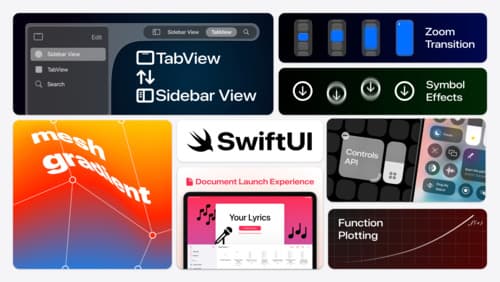
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
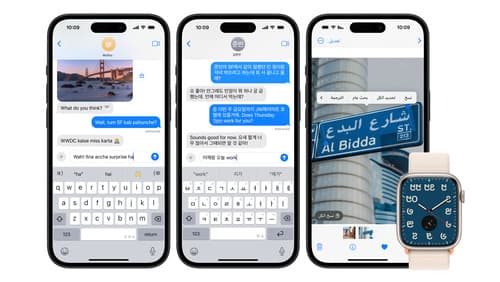
Build multilingual-ready apps
Ensure your app works properly and effectively for multilingual users. Learn best practices for text input, display, search, and formatting. Get details on typing in multiple languages without switching between keyboards. And find out how the latest advances in the String Catalog can make localization even easier.
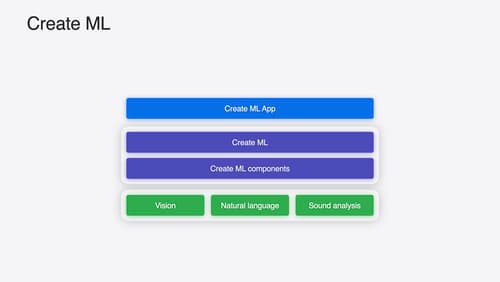
What’s new in Create ML
Explore updates to Create ML, including interactive data source previews and a new template for building object tracking models for visionOS apps. We’ll also cover important framework improvements, including new time-series forecasting and classification APIs.