best way to move to strict concurrency
Generated on 11/13/2024
1 search
To move to strict concurrency in Swift, especially with the transition to Swift 6, you can follow a structured approach as outlined in the WWDC sessions. Here are some key steps and considerations:
-
Enable Complete Concurrency Checking: Start by enabling complete concurrency checking in your app's build settings. This will allow the Swift compiler to emit warnings about code that may not be concurrency safe. It's recommended to begin with simpler targets, such as the UI layer, which often runs on the main thread and uses APIs like SwiftUI or UIKit that are main-thread safe. This approach is discussed in the session Migrate your app to Swift 6.
-
Adopt Swift 6 Language Mode: Swift 6 introduces data race safety by default, turning potential data race issues into compile-time errors. This significantly improves app security and reduces debugging time. You can adopt this mode module by module, allowing for incremental migration. This is covered in the session What’s new in Swift.
-
Use Actors and Sendable: Swift concurrency is designed around data isolation mechanisms, such as actors for protecting mutable state and the
Sendable
protocol for safe data sharing. These tools help prevent data races by ensuring that data is accessed safely across different threads. -
Utilize Low-Level Synchronization Primitives: Swift 6 introduces new low-level primitives like atomics and mutexes for synchronization. These can be used for efficient, lock-free implementations and ensure mutually exclusive access to shared resources. This is detailed in the session What’s new in Swift.
-
Incremental Strategy: When migrating, it's advisable to follow an incremental strategy. Enable concurrency checking and Swift 6 features in one part of your app, address any warnings or errors, and then move on to other parts. Avoid mixing significant refactoring with enabling data race safety to manage changes effectively.
By following these steps, you can transition your app to strict concurrency in Swift 6, ensuring data race safety and improving the overall quality and performance of your app.
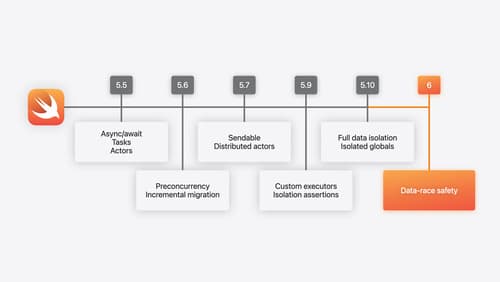
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
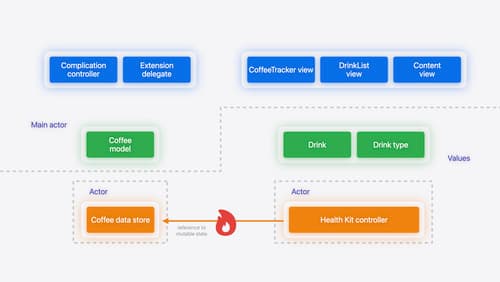
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.