how do I convert a Decimal to an Int?
Generated on 7/30/2024
1 search
To convert a Decimal
to an Int
in Swift, you can use the NSDecimalNumber
class, which provides a method to convert to an integer. Here is an example:
import Foundation
let decimalNumber = Decimal(10.5)
let intValue = NSDecimalNumber(decimal: decimalNumber).intValue
print(intValue) // Output: 10
This code snippet demonstrates how to convert a Decimal
to an Int
by first creating an NSDecimalNumber
from the Decimal
and then using the intValue
property to get the integer value.
For more detailed information on handling types in Swift, you might find the session Consume noncopyable types in Swift useful.
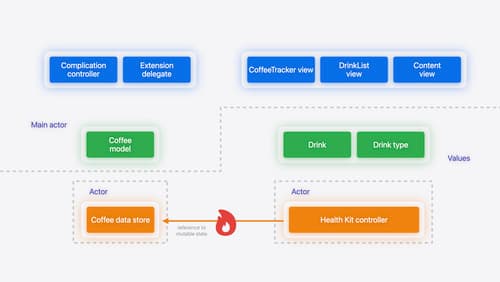
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
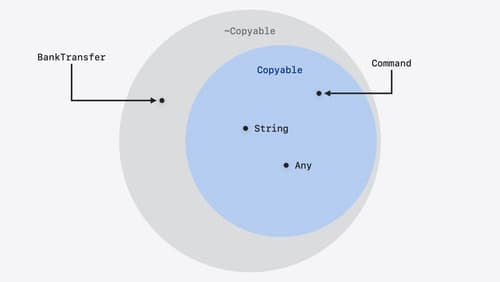
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.
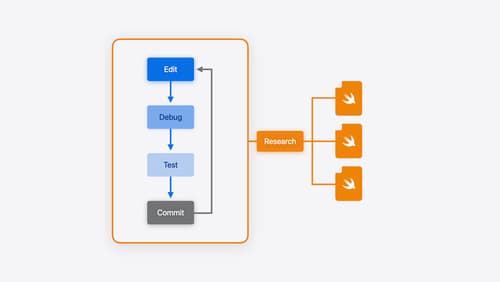
Xcode essentials
Edit, debug, commit, repeat. Explore the suite of tools in Xcode that help you iterate quickly when developing apps. Discover tips and tricks to help optimize and boost your development workflow.
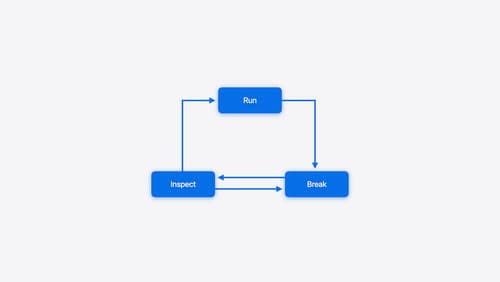
Run, Break, Inspect: Explore effective debugging in LLDB
Learn how to use LLDB to explore and debug codebases. We’ll show you how to make the most of crashlogs and backtraces, and how to supercharge breakpoints with actions and complex stop conditions. We’ll also explore how the “p” command and the latest features in Swift 6 can enhance your debugging experience.