what is difference between struct and class
Asked on 07/30/2024
1 search
In Swift, the primary difference between structs and classes lies in their storage and semantics:
-
Storage:
- Structs use inline storage. This means that the data for a struct is stored directly in the variable that holds it. When you copy a struct, you copy all of its data.
- Classes use out of line storage. This means that the data for a class is stored separately, and the variable holds a reference (pointer) to that data. When you copy a class, you copy the reference, not the actual data.
-
Semantics:
- Structs have value semantics. When you copy a struct, you get a completely independent copy of the data. Changes to one copy do not affect the other.
- Classes have reference semantics. When you copy a class, you get another reference to the same data. Changes to the data through one reference are visible through all references.
-
Performance Implications:
- Structs avoid heap allocation and can be more efficient for small data types because they are stored inline. However, copying large structs can be costly because all data is duplicated.
- Classes can be more efficient for large data types because they avoid copying the data itself, only the reference. However, they involve heap allocation and reference counting, which can introduce overhead.
-
Use Cases:
- Use structs when you need value semantics, such as for small, immutable data types or when you want to ensure that copies are independent.
- Use classes when you need reference semantics, such as for shared, mutable state or when you need to use inheritance.
For more detailed information, you can refer to the following sessions from WWDC:
Relevant Sessions
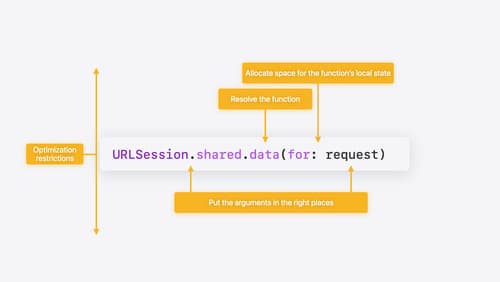
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
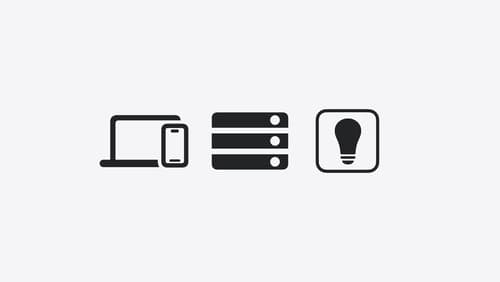
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
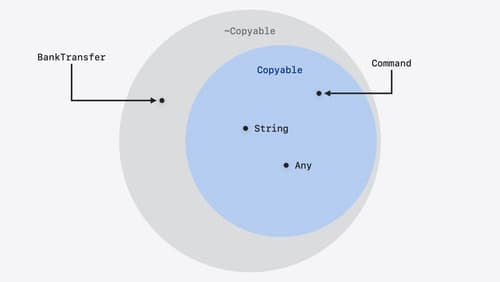
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.