How do I can define a text field in SwiftUI with text wrap and keyboardline breaks with dismissing the keyboard?
Generated on 7/30/2024
1 search
To define a text field in SwiftUI with text wrapping, keyboard line breaks, and the ability to dismiss the keyboard, you can use the following approach:
- Text Wrapping: Use a
TextEditor
instead of aTextField
for multi-line text input, which inherently supports text wrapping. - Keyboard Line Breaks:
TextEditor
also supports keyboard line breaks. - Dismissing the Keyboard: You can add a gesture recognizer to dismiss the keyboard when tapping outside the text editor.
Here's a sample implementation:
import SwiftUI
struct ContentView: View {
@State private var text: String = ""
var body: some View {
VStack {
TextEditor(text: $text)
.padding()
.background(Color.gray.opacity(0.2))
.cornerRadius(8)
.frame(height: 200)
.onTapGesture {
// Dismiss the keyboard when tapping outside the TextEditor
UIApplication.shared.sendAction(#selector(UIResponder.resignFirstResponder), to: nil, from: nil, for: nil)
}
}
.padding()
.onTapGesture {
// Dismiss the keyboard when tapping outside the VStack
UIApplication.shared.sendAction(#selector(UIResponder.resignFirstResponder), to: nil, from: nil, for: nil)
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Relevant Sessions
- What’s new in SwiftUI (15:06): This session discusses new features in SwiftUI, including programmatic access to and control of text selection within text editing controls.
- Build multilingual-ready apps (03:50): This session covers handling keyboard interactions and layout guides, which can be useful for customizing the placement of views in relation to the keyboard.
These sessions provide additional context and enhancements that can be applied to text fields and text editors in SwiftUI.
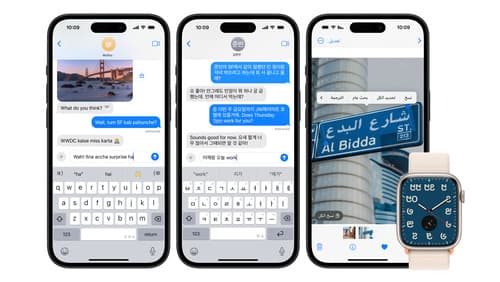
Build multilingual-ready apps
Ensure your app works properly and effectively for multilingual users. Learn best practices for text input, display, search, and formatting. Get details on typing in multiple languages without switching between keyboards. And find out how the latest advances in the String Catalog can make localization even easier.
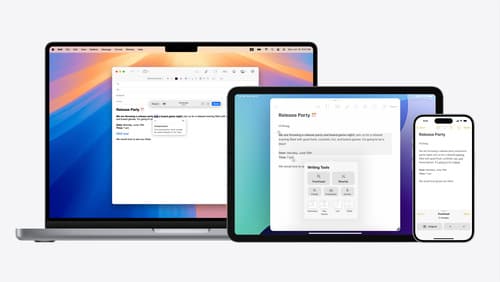
Get started with Writing Tools
Learn how Writing Tools help users proofread, rewrite, and transform text in your app. Get the details on how Writing Tools interact with your app so users can refine what they have written in any text view. Understand how text is retrieved and processed, and how to support Writing Tools in custom text views.
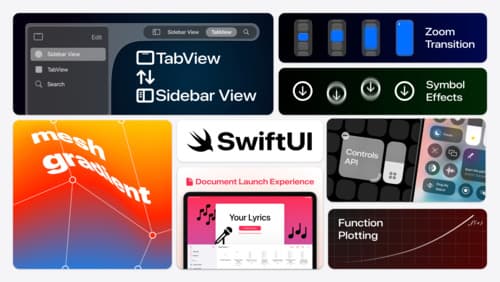
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
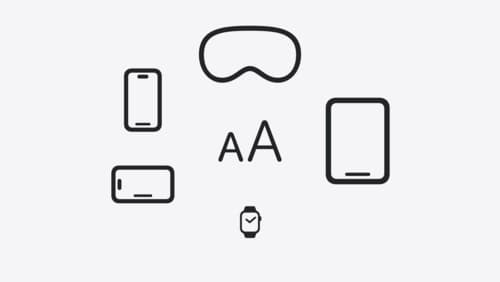
Get started with Dynamic Type
Dynamic Type lets people choose their preferred text size across the system and all of their apps. To help you get started supporting Dynamic Type, we’ll cover the fundamentals: How it works, how to find issues with scaling text in your app, and how to take practical steps using SwiftUI and UIKit to create a great Dynamic Type experience. We’ll also show how you can best use the Large Content Viewer to make navigation controls accessible to everyone.